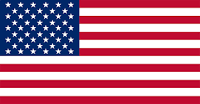
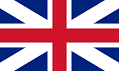
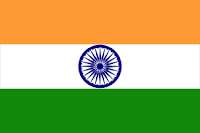
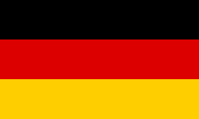
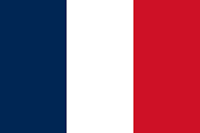
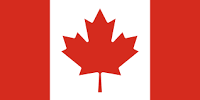
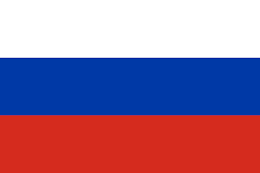
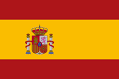
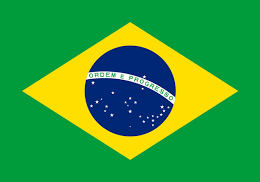
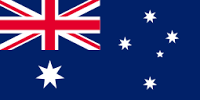
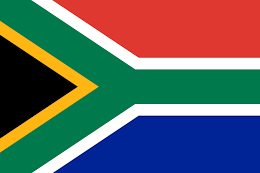
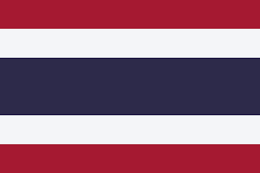
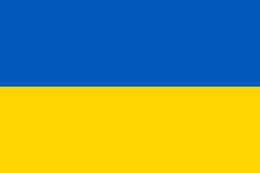
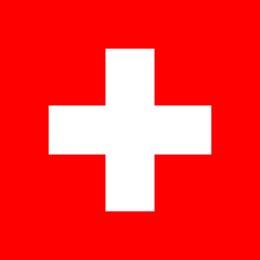
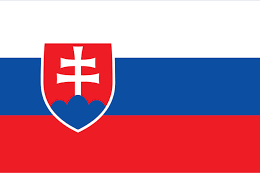
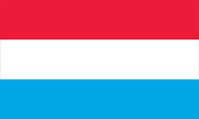
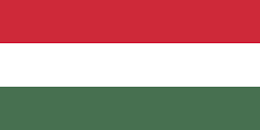
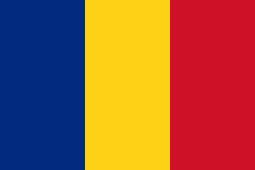
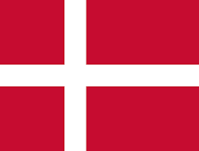
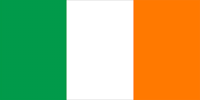
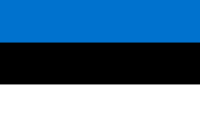
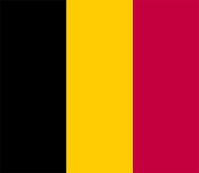
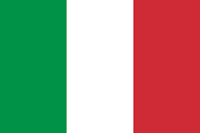
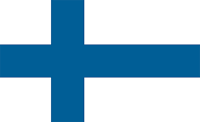
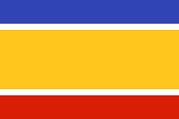
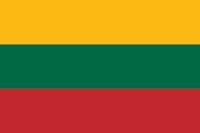
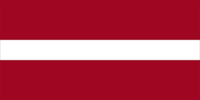
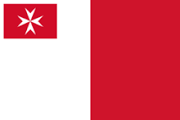
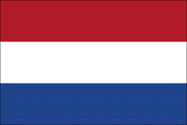
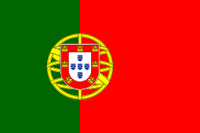
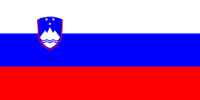
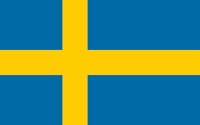
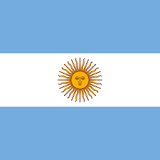
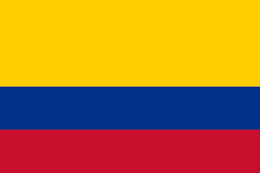
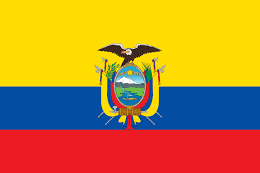
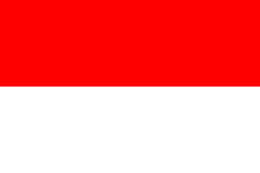
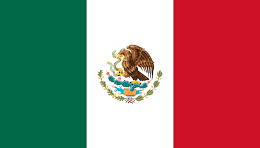
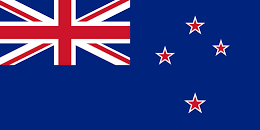
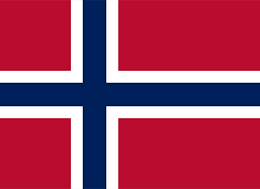
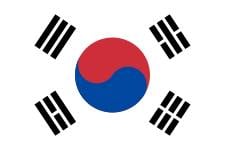
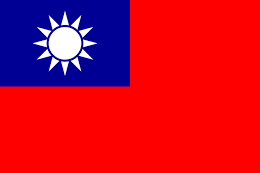
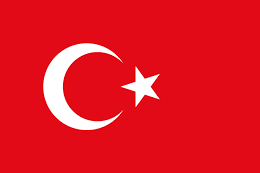
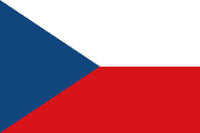
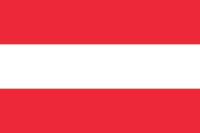
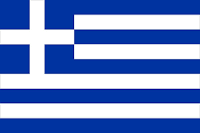
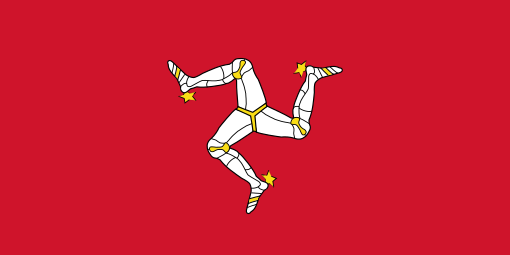
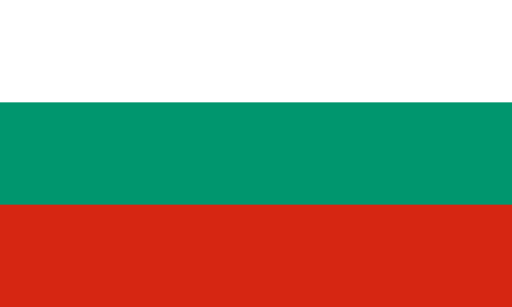
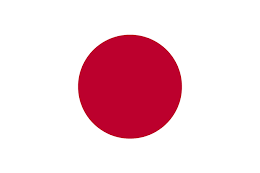
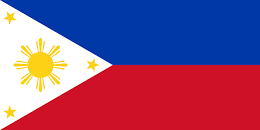
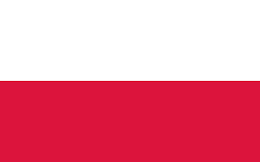

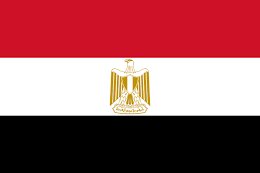
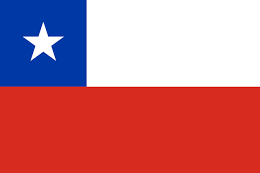
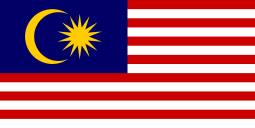
XAML is also known as Extensible Application Markup Language. XAML is a generic language like XML and can be used for multiple purposes and in the WPF and Silverlight applications. XAML is majorly used to declaratively design user interfaces.
In this article by Abhishek Shukla, author of the book Blend for Visual Studio 2012 by Example Beginner's Guide, we had a look at the various layout controls and at how to use them in our application. In this article, we will have a look at the following topics:
(For more resources related to this topic, see here.)
An important point to note here is that almost everything that we can do using XAML can also be done using C#. The following is the XAML and C# code to accomplish the same task of adding a rectangle within a canvas. In the XAML code, a canvas is created and an instance of a rectangle is created, which is placed inside the canvas:
<Canvas> <Rectangle Height="100" Width="250" Fill="AliceBlue" StrokeThickness="5" Stroke="Black" /> </Canvas>
Canvas layoutRoot = new Canvas(); Rectangle rectangle = new Rectangle(); rectangle.Height = 100; rectangle.Width = 250; rectangle.Fill = Brushes.AliceBlue; rectangle.StrokeThickness = 5; rectangle.Stroke = Brushes.Black; layoutRoot.Children.Add(rectangle); AddChild(layoutRoot);
We can clearly see why XAML is the preferred choice to define UI elements—XAML code is shorter, more readable, and has all the advantages that a declarative language provides. Another major advantage of working with XAML rather than C# is instant design feedback. As we type XAML code, we see the changes on the art board instantly. Whereas in the case of C#, we have to run the application to see the changes. Thanks to XAML, the process of creating a UI is now more like visual design than code development.
When we drag and drop an element or draw an element on the art board, Blend generates XAML in the background. This is helpful as we do not need to hand-code XAML as we would have to when working with text-editing tools.
Generally, the order of attaching properties and event handlers is performed based on the order in which they are defined in the object element. However, this should not matter, ideally, because, as per the design guidelines, the classes should allow properties and event handlers to be specified in any order.
Wherever we create a UI, we should use XAML, and whatever relates to data should be processed in code. XAML is great for UIs that may have logic, but XAML is not intended to process data, which should be prepared in code and posted to the UI for displaying purposes. It is data processing that XAML is not designed for.
Each element in XAML maps to an instance of a .NET class, and the name of the element is exactly the same as the class. For example, the <Button> element in XAML is an instruction to create an instance of the Button class. XAML specifications define the rules to map the namespaces, types, events, and properties of object-oriented languages to XML namespaces.
Perform the following steps and take a look at the XAML namespaces after creating a WPF application:
<Window x_Class="Chapter03.MainWindow" Title="MainWindow" Height="350" Width="525">
http://schemas.microsoft.com/winfx/2006/xaml/presentation is is the default namespace. The default namespace allows us to add elements to the page without specifying any prefix. So, all other namespaces defined must have a unique prefix for reference. For example, the namespace http://schemas.microsoft.com/winfx/2006/xaml is defined with the prefix :x. The prefix that is mapped to the schema allows us to reference this namespace just using the prefix instead of the full-schema namespace.
<Rectangle x_Name="someRectangle" Fill="AliceBlue"/>
We had a look at the XAML namespaces that we use when we create a WPF application.
In this section, we will add another namespace apart from the default namespace:
Prefix is the XML prefix we want to use in our XAML to represent that namespace. For example, the XAML namespace uses the :x prefix.
Namespace is the fully qualified .NET namespace.
AssemblyName is the assembly where the type is declared, and this assembly could be the current project assembly or a referenced assembly.
To create an instance of an object we would have to use this namespace prefix as <local:MyObj></local:MyObj>
We saw how we can add more namespaces in XAML apart from the ones present by default and how we can use them to create objects.
In XAML, it is not mandatory to add a name to every element, but we might want to name some of the XAML elements that we want to access in the code or XAML. We can change properties or attach the event handler to, or detach it from, elements on the fly.
We can set the name property by hand-coding in XAML or setting it in the properties window. This is shown in the following screenshot:
The x:Class attribute in XAML references the code-behind class for XAML. You would notice the x:, which means that the Class attribute comes from the XAML namespace, as discussed earlier. The value of the attribute references the MainWindow class in the Chapter03 namespace. If we go to the MainWindow.xaml.cs file, we can see the partial class defined there. The code-behind class is where we can put C# (or VB) code for the implementation of event handlers and other application logic. As we discussed earlier, it is technically possible to create all of the XAML elements in code, but that bypasses the advantages of having XAML.
So, as these two are partial classes, they are compiled into one class. So, as C# and XAML are equivalent and both these are partial classes, they are compiled into the same IL.
public MainWindow() { InitializeComponent(); LayoutRoot.Background = Brushes.Green; }
We accessed the element defined in XAML by its name.
The content of a XAML element is the value that we can simply put between the tags without any specific references. For example, we can set the content of a button as follows:
<Button Content="Some text" /> or <Button > Some text </Button>
The default properties are specified in the help file. So, all we need to do is press F1 on any of our controls to see what the value is.
We can express the properties of an element as an XML attribute.
In this section, instead of dragging and dropping controls, we will add XAML code:
<Grid x_Name="LayoutRoot"> <TextBlock Text="00:00" Height="170" Margin="49,32,38,116" Width="429" FontSize="48"/> <Button Content="Start" Height="50" Margin="49,220,342,49" Width="125"/> <Button Content="Stop" Height="50" Margin="203,220,188,49" Width="125"/> <Button Content="Reset" Height="50" Margin="353,220,38,49" Width="125"/> </Grid>
We added elements in XAML with properties as XML attributes.
We will define a gradient background for the grid, which is a complex property. Notice that the code in the next section sets the background property of the grid using a different type converter this time. Instead of a string-to-brush converter, the LinearGradientBrush to brush type converter would be used.
<Grid x_Name="LayoutRoot"> <Grid.Background> <LinearGradientBrush EndPoint="1,1" StartPoint="0,0"> <GradientStop Color="Black"/> <GradientStop Color="#FF27EC07" Offset="1"/> </LinearGradientBrush> </Grid.Background>
The following is the output of the preceding code:
Using <!-- --> tags, we can add comments in XAML just as we add comments in XML. Comments are really useful when we have complex XAML with lots of elements and complex layouts:
<!-- TextBlock to show the timer -->
We would like all the buttons in our application to look the same, and we can achieve this using styles. Here, we will just see how styles can be defined and used in XAML.
We will define a style as a static resource in the window. We can move all the properties we define for each button to that style.
Add a new <Window.Resources> tag in XAML, and then add the code for the style, as shown here:
<Window.Resources> <Style TargetType="Button" x_Key="MyButtonStyle"> <Setter Property="Height" Value="50" /> <Setter Property="Width" Value="125" /> <Setter Property="Margin" Value="0,10" /> <Setter Property="FontSize" Value="18"/> <Setter Property="FontWeight" Value="Bold" /> <Setter Property="Background" Value="Black" /> <Setter Property="Foreground" Value="Green" /> </Style> </Window.Resources>
We defined a few properties on style that are worth noting:
We defined a style for a button in the window.
Let's use the style that we defined in the previous section. All UI controls have a style property (from the FrameworkElement base class).
<Button x_Name="BtnStart" Content="Start" Grid.Row="1" Grid.Column="0" Style="{StaticResource MyButtonStyle}"/>
The following is the output of the preceding code:
We used a style defined for a button.
This article gave a brief overview of XAML, which helps you to start designing your applications in Expression Blend. However, if you wish know more about XAML, you could visit the following MSDN links and go through the various XAML specifications and details:
Q1. How can we find out the default property of a control?
Q2. Is it possible to create a custom type converter?
We had a look at the basics of XAML, including namespaces, elements, and properties. With this introduction, you can now hand-edit XAML, where necessary, and this will allow you to tweak the output of Expression Blend or even hand-code an entire UI if you are more comfortable with that.
Further resources on this subject: