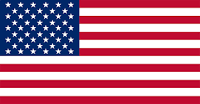
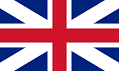
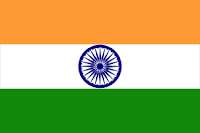
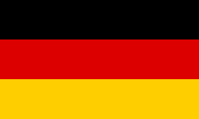
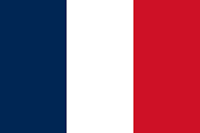
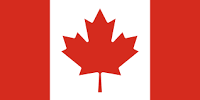
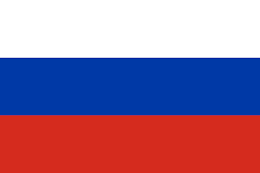
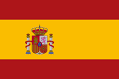
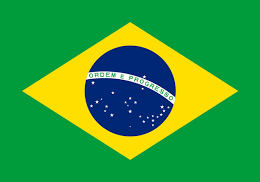
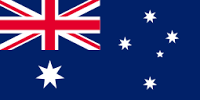
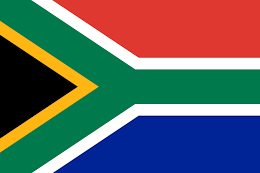
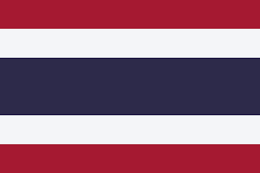
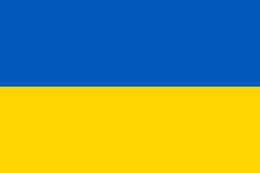
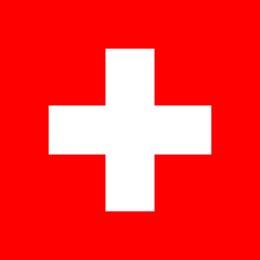
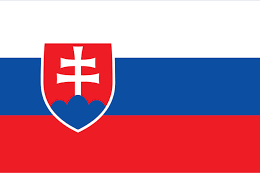
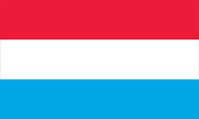
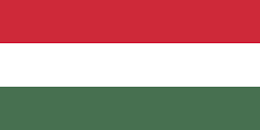
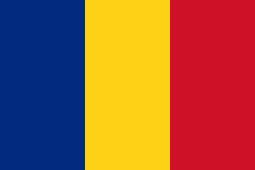
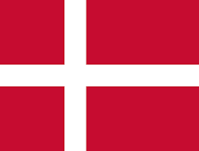
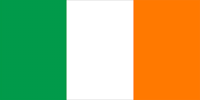
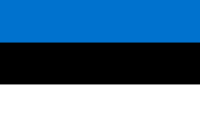
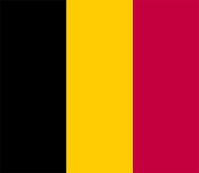
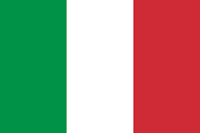
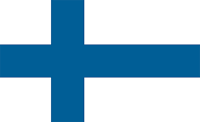
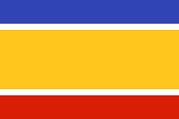
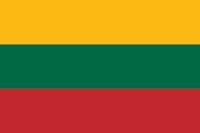
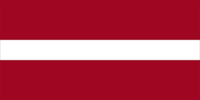
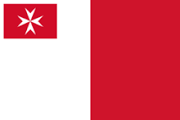
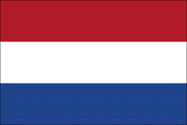
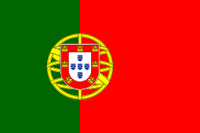
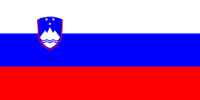
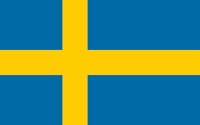
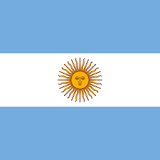
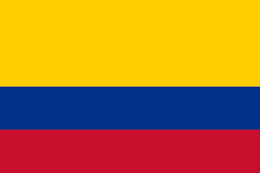
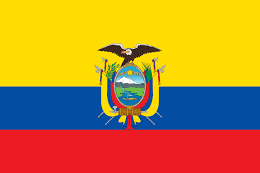
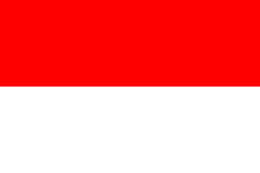
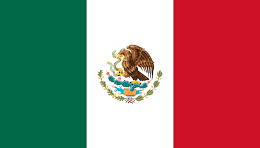
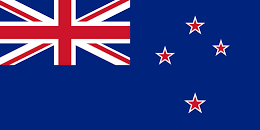
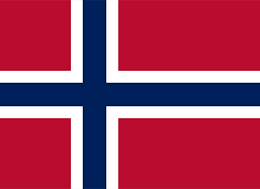
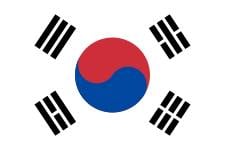
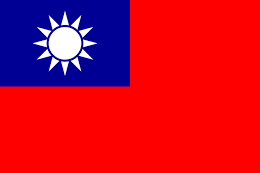
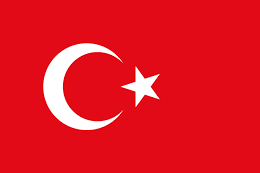
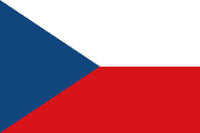
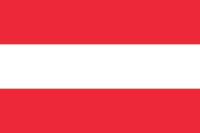
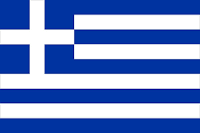
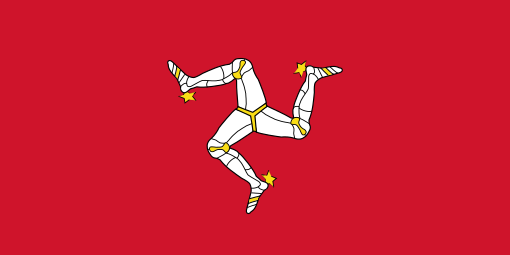
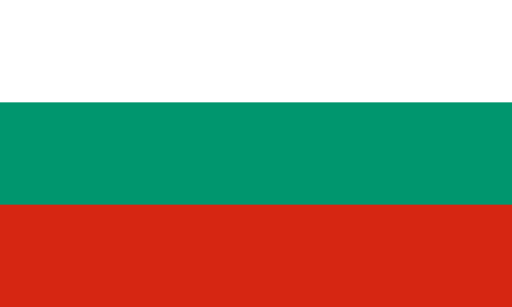
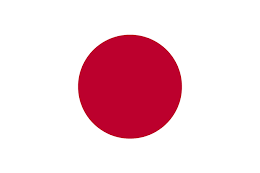
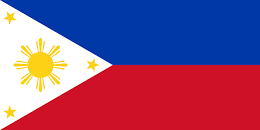
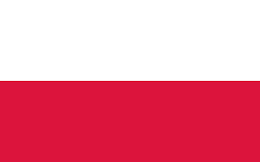

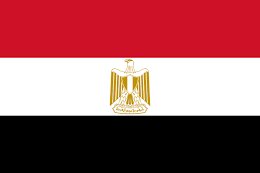
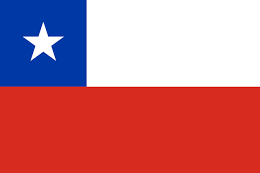
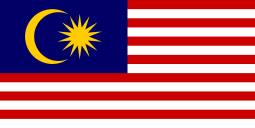
Read more about this book |
(For more resources on jQuery, see here.)
Before we get started, there is another little thing worth mentioning: provide many different opportunities to introduce new concepts and ideas, even while keeping the complexity of the whole plugin at a minimum.
We can now go on to create our plugin, starting with basic functionalities, and subsequently adjusting its goals. We will add new, improved functionalities that, however, do not make the whole code look too difficult to understand—even after some time or for someone who's just starting out with jQuery.
A lot has been said about tooltip plugins, but it's worth repeating the most important points with particular regard to the way tooltips are supposed to work, and how we want our tooltip to behave.
First of all, we might want to get an idea of what tooltips look like and a sample of what we will accomplish by the end of this article. Here is an example:
Also, with some more work and proper application of effects, images, and other relatively advanced techniques, we can also obtain something more complex and nicer looking, thus giving the user the chance to specify the style and behavior for the tooltip, as follows:
The idea is actually very simple. The elements we have selected will trigger an event every time we hover the mouse pointer over them.
The tooltip will then pop out, right at the mouse cursor position, retrieving the text portion from the title attribute of the said element.
Finally, whenever we move the mouse over the same element, the plugin will move and follow the mouse cursor until it goes off the boundaries of the element.
The first problem we have to face is, of course, how to make the tooltip appear in the right position.
It would be no trouble at all if we just had to make some text, image, or anything else show up. We've done it many times and it's no problem at all—just make their positioning absolute and set the right top and side distances.
However, we need to take into account the fact that we don't know exactly where the mouse cursor might be and, as such, we need to calculate distances based upon the mouse cursor position itself.
So, how can we do it? It's simple enough; we can use some of the JavaScript event properties to obtain the position. Unfortunately, Internet Explorer always tries to put a spoke in our wheel.
In fact, the magnificent browser does not (according to this table, which is quite accurate: http://www.quirksmode.org/dom/w3c_cssom.html#mousepos) support pageX and pageY, which would normally return the mouse coordinates relative to the document.
So we need to think about a workaround for Internet Explorer, as jQuery (from version 1.0.4 onwards) does not normalize some of the event properties according to W3C standards (http://api.jquery.com/category/events/event-object/).
The following diagram (also provided in the " target="_blank">code bundle) should clarify what the visible viewport is (that is, the browser window—the red box):
Whenever we scroll down, different parts of the document (blue) are shown through the browser window and hidden due to space constraints. The scroll height (green) is the part of the document currently not displayed.
Suppose we have a page with some text written in, which also contains a few links to both internal pages (that is, pages on the same server) and external websites.
We are presented with different choices in terms of which elements to apply the tooltip to (referring to links as an example, but they apply to any kind of element as well), as follows:
We can easily combine the first three conditions with the others (and with themselves) using CSS selectors appropriately. For example:
As for internal and external pages, we have to work with jQuery selectors instead.
Although jQuery makes it easy to select elements using standard CSS selectors, as well as some other selectors, jQuery's own selectors are the ones that help the developer to write and read code. Examples of custom selectors are :odd, :animated, and so on.
jQuery also lets you create your own selectors!
// definition
$.expr[':'].customselector = function(object, index,
properties, list) {
// code goes here
};
// call
$("a:customselector")
$.expr[':'].external = function(object) {
if(object.hostname) // is defined
return(object.hostname != location.hostname);
else return false;
};
$.expr[':'].sample = function(object) {
alert('$(obj).attr(): ' + $(object).attr("href") +
'obj.href: ' + object.href);
};
We have slowly created different parts of the plugin, which we need to merge in order to create a working piece of code that actually makes tooltips visible.
So far we have understood how positioning works and how we can easily place an element in a determined position.
Also, we have found out we can create our own jQuery selectors, and have developed a simple yet useful custom selector with which we are able to select links pointing to either internal or external pages. It needs to be placed at the top of the code, inside the closure, as we will make use of the dollar symbol ($) and it may conflict with other software.