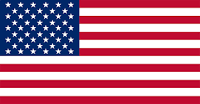
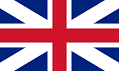
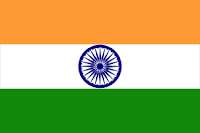
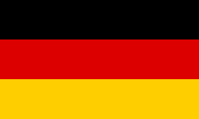
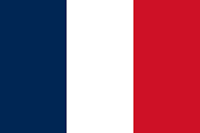
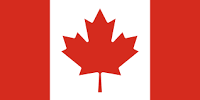
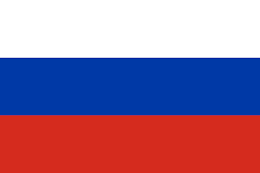
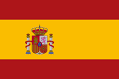
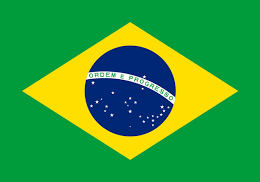
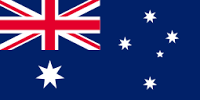
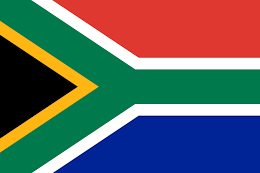
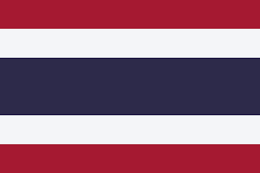
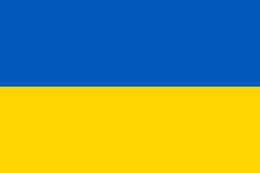
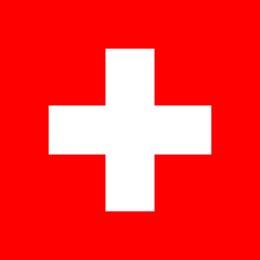
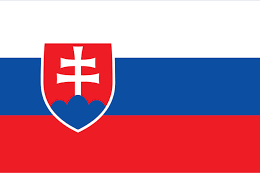
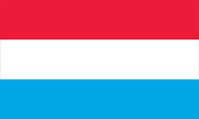
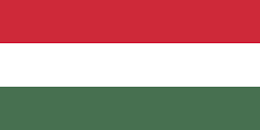
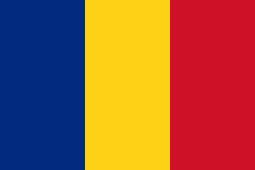
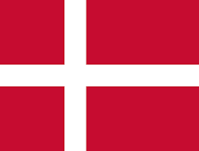
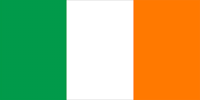
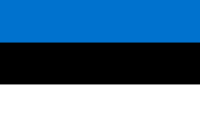
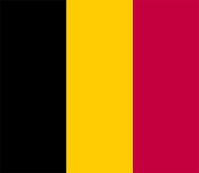
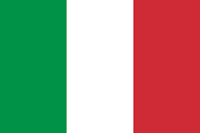
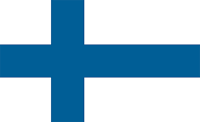
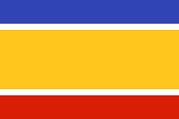
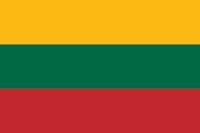
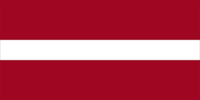
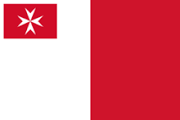
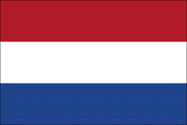
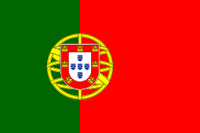
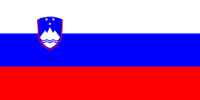
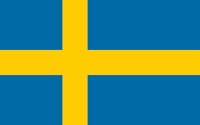
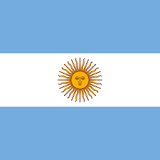
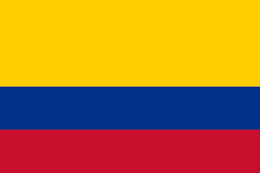
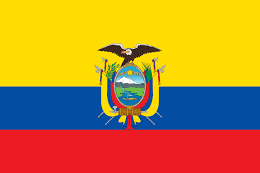
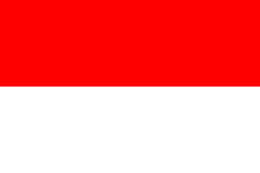
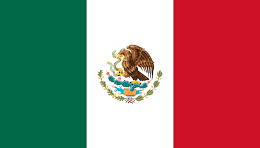
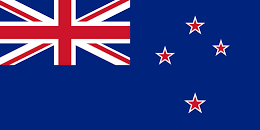
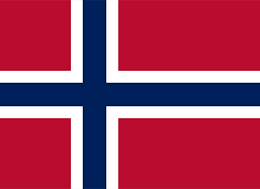
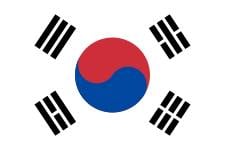
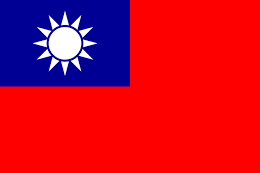
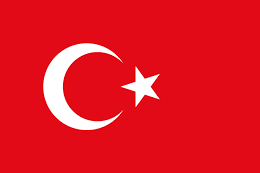
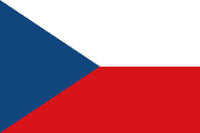
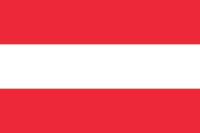
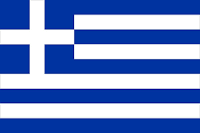
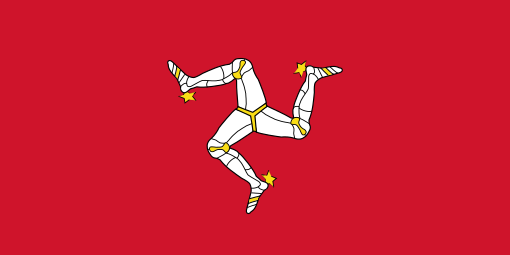
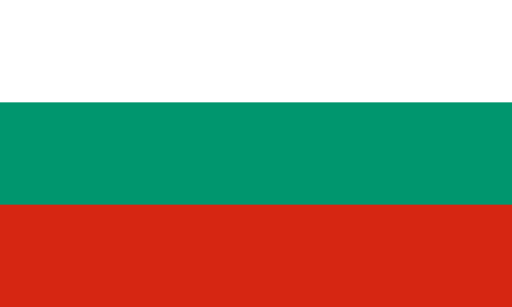
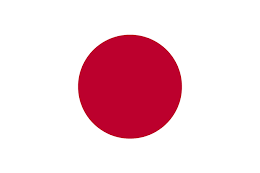
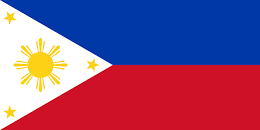
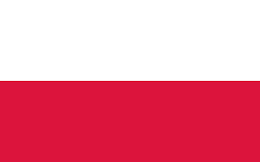

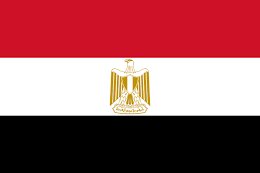
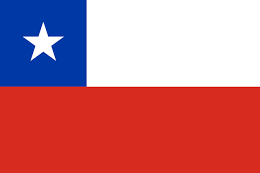
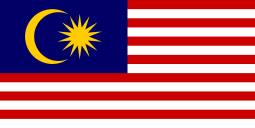
In this article by Raghavendra Prasad MG, author of the book Learning Selenium Testing Tools, Third Edition you will be introduced to the Selenium IDE WebDriver by demonstrating its installation, basic features, its advanced features, and implementation of automation framework with programming language basics required for automation.
Automation being a key point of success of any software organization, everybody is looking at the freeware and huge community supported tool like Selenium.
Anybody who is willing to learn and work on automation with Selenium has an opportunity to learn the tool from basic to advanced stage with this book and the book would become a life time reference for the reader.
(For more resources related to this topic, see here.)
Following are the key features of the book:
There are a lot of things you will learn from the book. A few of them are mentioned as follows:
The book is for manual testers. Even any software professionals and the ones who wants to make their carrier in Selenium automation testing can use this book. This book also helps automation testers / automation architects who want to build or implement the automation / automation frameworks on Selenium automation tool.
The book covers the following major topics:
Selenium IDE is a Firefox add-on developed originally by Shinya Kasatani as a way to use the original Selenium Core code without having to copy Selenium Core onto the server. Selenium Core is the key JavaScript modules that allows Selenium to drive the browser. It has been developed using JavaScript so that it can interact with the DOM (Document Object Model) using native JavaScript calls.
Selenium IDE has been developed to allow testers and developers to record their actions as they follow the workflow that they need to test.
Locators shows how we can find elements on the page to be used in our tests. We will use XPath, CSS, link text, and ID to find elements on the page so that we can interact with them.
Locators allow us to find elements on a page that can be used in our tests. In the last chapter we managed to work against a page which had decent locators. In HTML, it is seen as a good practice to make sure that every element you need to interact with has an ID attribute and a name attribute. Unfortunately, following best practices can be extremely difficult, especially when building the HTML dynamically on the server before sending it back to the browser.
Following are the locators used in Selenium IDE:
Locators |
Description |
Example |
ID |
This element identifies an ID attribute on the page |
id=inputButton |
name |
This element identifies name attribute on the page |
name=buttonFind |
link |
This element identifies links by the text |
link=index |
XPath |
This element identifies by XPath |
xpath=//div[@class='classname'] |
CSS |
This element identifies by CSS |
css=#divinthecenter |
DOM |
This element identifies by DOM |
dom=document.getElementById("inputButton") |
The primary feature of the Selenium WebDriver is the integration of the WebDriver API and its design to provide a simpler, more concise programming interface in addition to addressing some limitations in the Selenium-RC API. Selenium WebDriver was developed to better support dynamic web pages where elements of a page may change without the page itself being reloaded. WebDriver's goal is to supply a well designed object-oriented API that provides improved support for modern advanced web application testing problems.
When working with WebDriver on a web application, we will need to find elements on the page. This is the Core to being able to work. All the methods for performing actions to the web application, like typing and clicking require that we search the element first.
The first item that we are going to look at is finding an element by ID. Searching elements by ID is one of the easiest ways to find an element. We start with findElementByID(). This method is a helper method that sets an argument for a more generic findElement call. We will see now how we can use it in action. The method's signature looks like the following line of code:
findElementById(String using);
The using variable takes the ID of the element that you wish to look for. It will return a WebElement object that we can then work with.
We find an element on the page by using the findElementById() method that is on each of the Browser Driver classes. findElement calls will return a WebElement object that we can perform actions on.
Follow these steps to see how it works:
WebElement element = ((FindsById)driver). findElementById("verifybutton");
In this section of the article, we are going to have a look at how we can apply some best practices to tests. You will learn how to make maintainable test suites that will allow you to update tests in seconds. We will have a look at creating your own DSL so that people can see intent. We will create tests using the Page Object pattern.
FirefoxDriver is the easiest driver to use, since everything that we need to use is all bundled with the Java client bindings.
We do the basic task of loading the browser and type into the page as follows:
driver = new FirefoxDriver();
WebElement element = driver.findElement(By.id("nextBid"));
element.sendKeys("100");
import org.openqa.selenium.*; import org.openqa.selenium.firefox.*; import org.testng.annotations.*; public class TestChapter6 { WebDriver driver; @BeforeTest public void setUp(){ driver = new FirefoxDriver(); driver.get
("http://book.theautomatedtester.co.uk/chapter4"); } @AfterTest public void tearDown(){ driver.quit(); } @Test public void testExamples(){ WebElement element = driver.findElement(By.id("nextBid")); element.sendKeys("100"); } }
We are currently witnessing an explosion of mobile devices in the market. A lot of them are more powerful than your average computer was just over a decade ago. This means, that in addition to having nice clean, responsive, and functional desktop applications, we are starting to have to make sure the same basic functionality is available to mobile devices. We are going to be looking at how we can set up mobile devices to be used with Selenium WebDriver.
We will learn the following topics:
Selenium Grid is a version of Selenium that allows teams to set up a number of Selenium instances and then have one central point to send your Selenium commands to. This differs from what we saw in Selenium Remote WebDriver where we always had to explicitly say where the Selenium Server is as well as know what browsers that server can handle.
With Selenium Grid we just ask for a specific browser, and then the hub that is part of Selenium Grid will route all the Selenium commands through to the Remote Control you want.
We have understood and learnt what is Selenium and its evolutions from IDE to WebDriver and Grid as well.
In addition we have learnt how to identify the WebElements using WebDriver, and its design pattern and locators through WebDriver. And we learnt on the automation framework design and implementation, mobile application automation on Android and iOS. Finally we understood the concept of Selenium Grid.
Further resources on this subject: