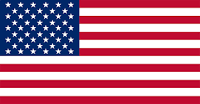
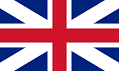
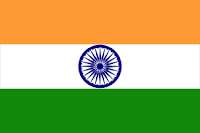
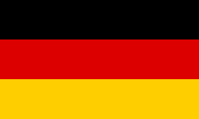
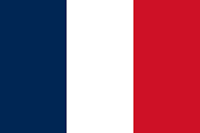
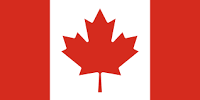
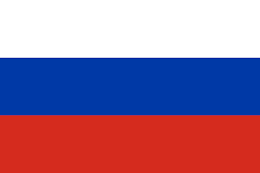
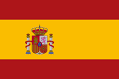
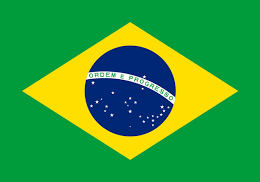
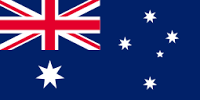
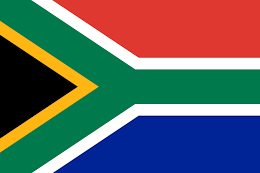
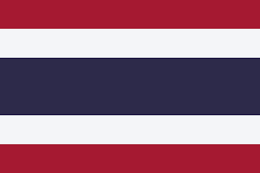
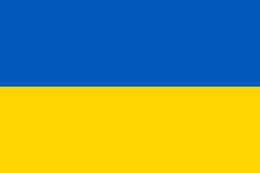
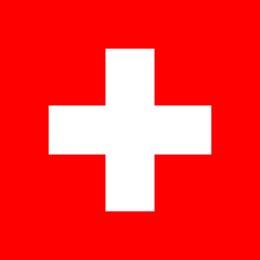
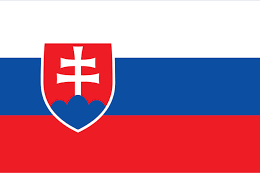
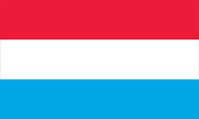
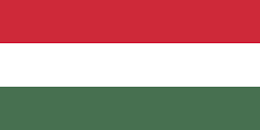
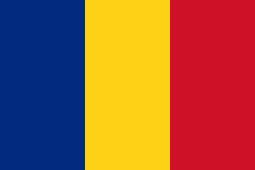
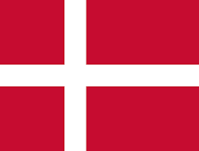
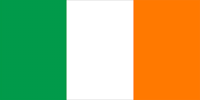
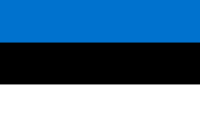
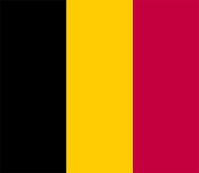
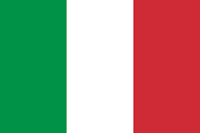
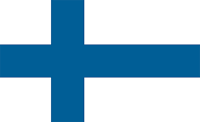
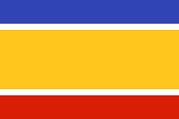
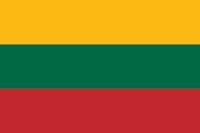
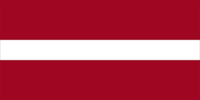
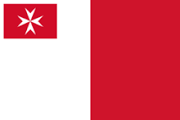
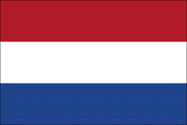
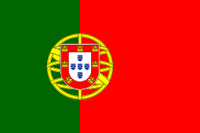
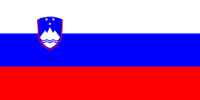
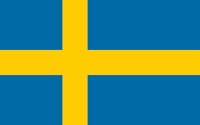
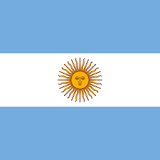
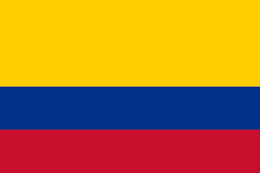
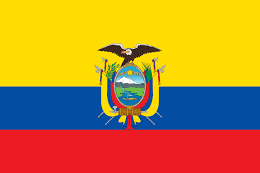
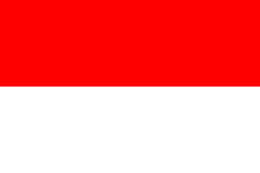
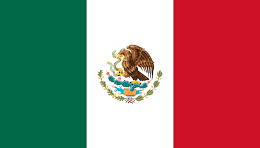
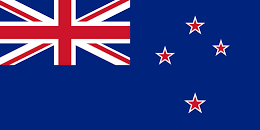
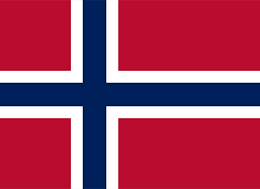
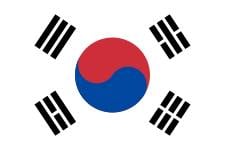
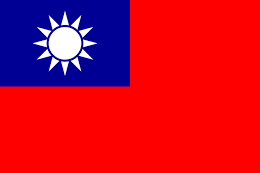
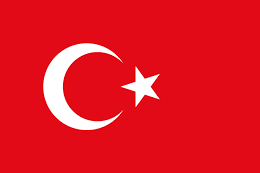
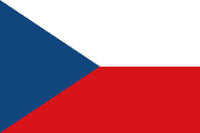
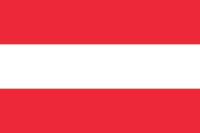
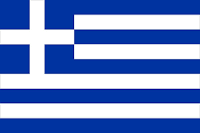
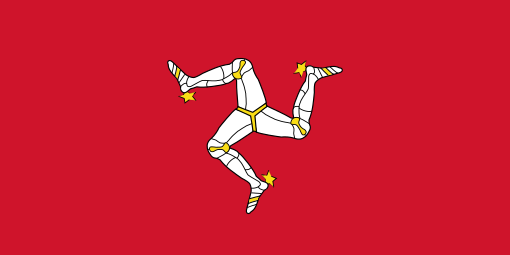
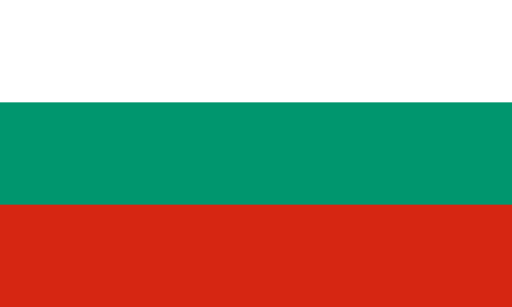
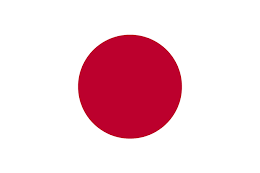
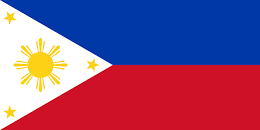
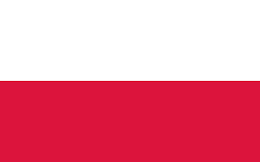

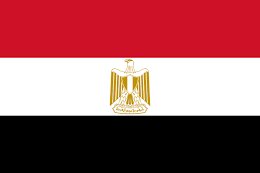
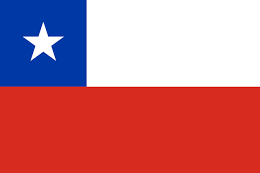
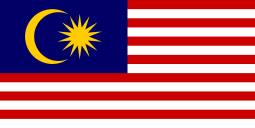
(For more resources related to this topic, see here.)
Handling specifc events is something everybody expects from an application. While JavaScript has its own event handling model, working with Dynamics CRM offers a different set of events that we can take advantage of.
The JavaScript event model, while it might work, is not supported, and defnitely not the approach you want to take when working within the context of Dynamics CRM.
Some of the most notable events and their counterparts in JavaScript are described in the following table:
Dynamics CRM 2011 |
JavaScript |
Description |
OnLoad |
onload |
This is a form event. Executes when a form is loaded. Most common use is to filter and hide elements on the form. |
OnSave |
onsubmit |
This is a form event. It executes when a form is saved. Most common use is to stop an operation from executing, as a result of a failed validation procedure. |
TabStateChange |
n/a |
This is a form event. It executes when the DisplayState of the tab changes. |
OnChange |
onchange |
This is a field specific event. It executes when tabbing out of a field where you've changed the value. Please note that there is no equivalent for onfocus and onblur. |
OnReadyStateComplete |
n/a |
This event indicates that the content of an IFrame has completed loading. |
Additional details on Dynamics CRM 2011 specifc events can be found on MSDN at http://msdn.microsoft.com/en-us/library/gg334481.aspx.
In this recipe, we will focus on executing a few operations triggered by the form load event. We can check the value of a specifc field on the form, and based on that we can decide to hide a tab, hide a field, and prepopulate a text field with a predefned value.
Just as with any of the previous recipes, you will need access to an environment, and permissions to make customizations. You should be a system administrator, a system customizer, or a custom role configured to allow you to perform the following operations.
For the purpose of this exercise, we will add to the Contact entity a new tab called "Special Customer", with some additional custom fields. We will also add an option set that we will check to determine if we hide or not the fields, as well as two new fields: one text field and one lookup field. So let's get started!
Observe the fact that I have ordered the three fields one on top of the other. The reason for this is because the default tab order in CRM is vertical and across. This way, when all the fields are visible, I can tab right from one to another.
function IsSpecialCustomer()
{
var _isSpecialSelection = null;
var _isSpecial = Xrm.Page.getAttribute("new_isspecialcustomer");
if(_isSpecial != null)
{
_isSpecialSelection = _isSpecial.getValue();
}
if(_isSpecialSelection == false)
{
// hide the Special Customer tab
Xrm.Page.ui.tabs.get("tab_5").setVisible(false);
// hide the Customer Classification field
Xrm.Page.ui.controls.get("new_customerclassification").
setVisible(false);
// hide the Partner field
Xrm.Page.ui.controls.get("new_partner").setVisible(false);
}
}
The whole idea of this script is not much different from what we have demonstrated in some of the previous recipes. Based on a set form value, we hide a tab and some fields. Where we capture the difference is where we set the script to execute. Working with scripts executing when the form loads gives us a whole new way of handling various scenarios.
In many scenarios, working with the form load events in conjunction with the other field events can potentially result in a very complex solution.
When debugging, always pay close attention to the type of event you associate your script function with.
See the Combining events recipe towards the end of this article for a more complex recipe detailing how to work with multiple events to achieve the expected result.
While working with the Form OnLoad event can help us format and arrange the user interface, working with the Form OnSave opens up a new door towards validation of user input and execution of business process amongst others.
Using the same solution we have worked on in the previous recipe, we will continue to demonstrate a few other aspects of working with the forms in Dynamics CRM 2011. In this recipe the focus is on the handling the Form OnSave event.
First off, in order to kick off this, we might want to verify a set of fields for a condition, or perform a calculation based on a formula. In order to simplify this process, we can just check a simple yes/no condition on a form.
Using the previously customized solution, we will be taking advantage of the Contact entity and the fields that we have already customized on that form. If you are starting with this recipe fresh, take the following step before delving into this recipe:
Using this field, if the answer is No, we will stop the save process.
function StopSave(context)
{
var _isSpecialSelection = null;
var _isSpecial = Xrm.Page.getAttribute("new_isspecialcustomer");
if(_isSpecial != null)
{
_isSpecialSelection = _isSpecial.getValue();
}
if(_isSpecialSelection == false)
{
alert("You cannot save your record while the Customer is not a
friend!");
context.getEventArgs().preventDefault();
}
}
While this recipe only describes in a very simplistic manner the way to stop a form from saving and closing, the possibilities here are immense. Think about what you can do on form save, and what you can achieve if a condition should be met in order to allow the form to be saved.
Starting a process instead of saving the form
Another good use for blocking the save and close form is to take a different path. Let's say we want to kick off a workfow when we block the save form. We can call from the previous function a new function as follows:
function launchWorkflow(dialogID, typeName, recordId)
{
var serverUri = Mscrm.CrmUri.create('/cs/dialog/rundialog.aspx');
window.showModalDialog(serverUri + '?DialogId=' + dialogID +
'&EntityName=' + typeName +
'&ObjectId=' + recordId, null, 'width=615,height=480,resizable=1,statu
s=1,scrollbars=1');
// Reload form
window.location.reload(true);
}
We pass to this function the following three parameters:
For more details on parameters see the following article on MSDN:
http://msdn.microsoft.com/en-us/library/gg309332.aspx
In this recipe we will drill down to a lower level. We have handled form events, and now it is time to handle field events. The following recipe will show you how to bring all these together and achieve exactly the result you need.
For the purpose of this recipe, let's focus on reusing the previous solution. We will check the value of a field, and act upon it.
In order to walkthrough this recipe, follow these steps:>
function ChangeEvent()
{
var _changeEventSelection = null;
var _isChanged = Xrm.Page.getAttribute("new_changeevent");
if(_isChanged != null)
{
_changeEventSelection = _isChanged.getValue();
}
if(_changeEventSelection == true)
{
alert("Change event is set to True");
// perform other actions here
}
else
{
alert("Change event is set to False");
}
}
Handling events at the field level, specifcally the OnSave event, allows us to dynamically execute various other functions. We can easily take advantage of this functionality to modify the form displayed to a user dynamically, based on a selection. Based on a field value, we can defne areas or field on the form to be hidden and shown.