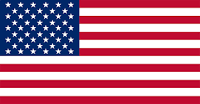
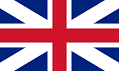
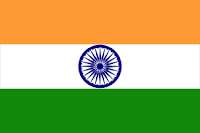
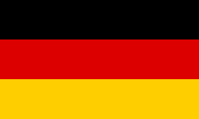
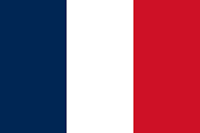
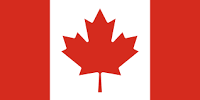
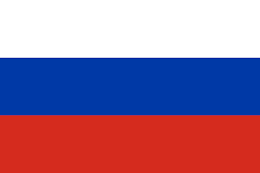
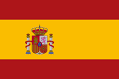
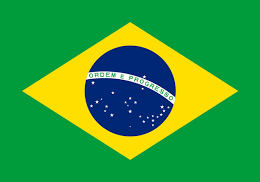
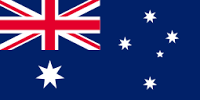
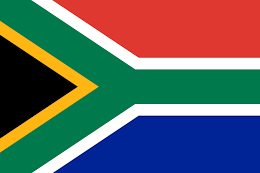
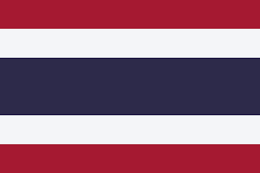
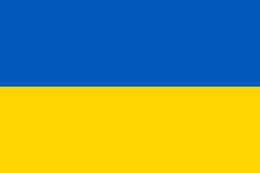
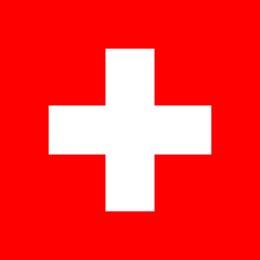
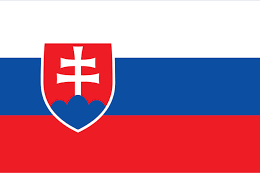
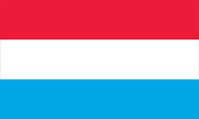
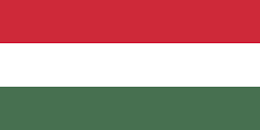
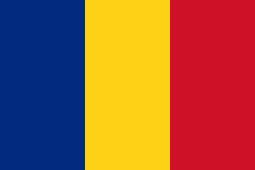
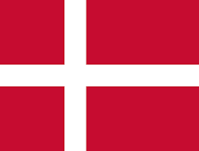
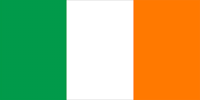
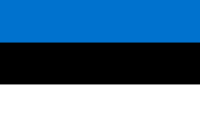
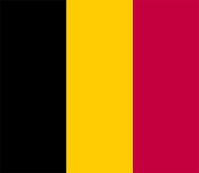
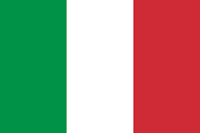
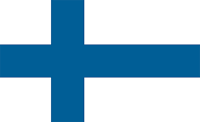
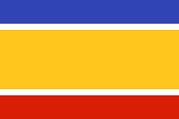
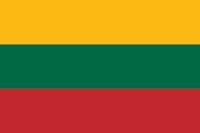
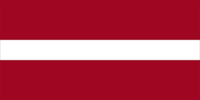
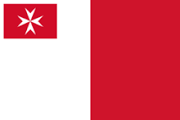
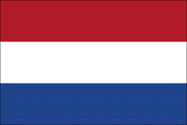
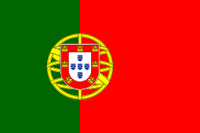
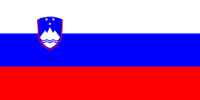
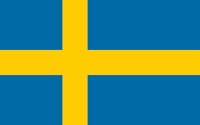
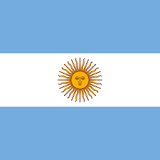
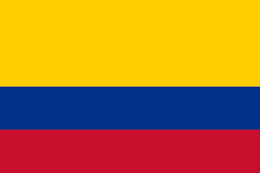
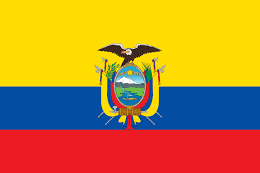
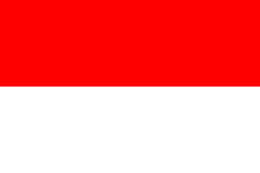
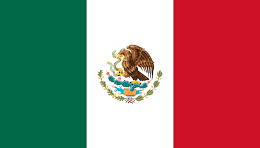
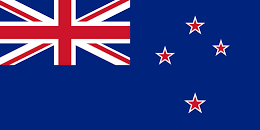
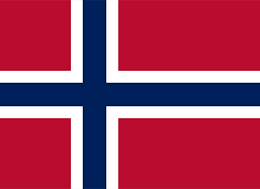
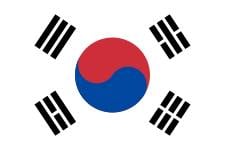
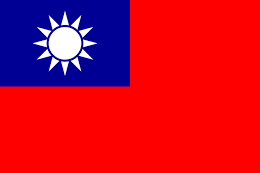
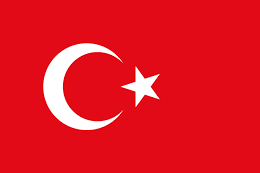
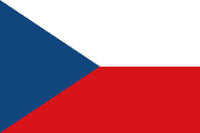
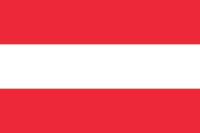
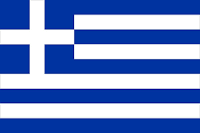
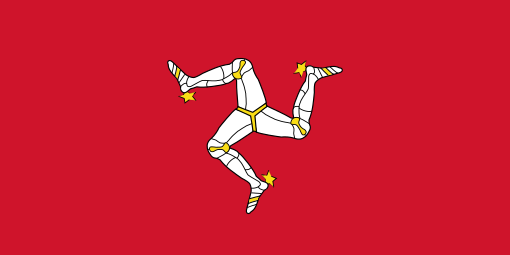
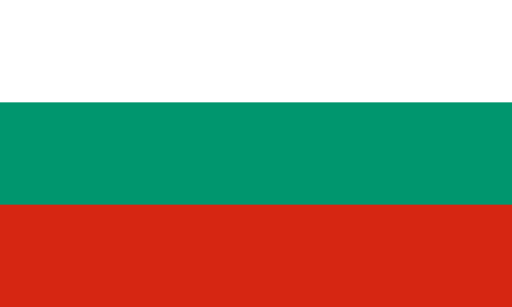
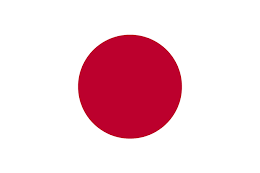
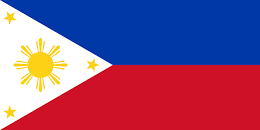
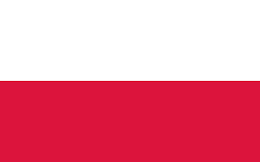

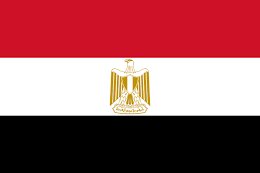
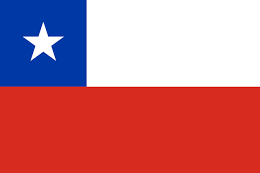
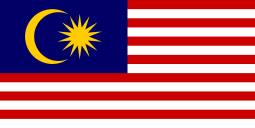
In this extract from Learning Object-Oriented Programming by Gaston Hillar, we will show you the benefits of thinking in an object-oriented way. From encapsulation and inheritance to polymorphism or object-overloading, we will hammer home the thought process that must be mastered in order to truly make the most of the object-oriented approach.
In this article, we will see how to generate blueprints for objects and we will design classes which include the attributes or fields that provide data for each instance. We will explore the different object-oriented approaches in different languages, such as Python, JavaScript, and C#.
Imagine that you want to draw and calculate the areas of four different rectangles. You will end up with four rectangles each with different widths, heights, and areas. Now imagine having a blueprint to simplify the process of drawing each different rectangle.
In object-oriented programming, a class is a blueprint or a template definition from which the objects are created. Classes are models that define the state and behavior of an object. After defining a class that determines the state and behavior of a rectangle, we can use it to generate objects that represent the state and behavior of each real-world rectangle.
Objects are also known as instances. For example, we can say each rectangle object is an instance of the rectangle class.
The following image shows four rectangle instances, with their widths and heights specified: Rectangle #1, Rectangle #2, Rectangle #3, and Rectangle #4. We can use a rectangle class as a blueprint to generate the four different rectangle instances. It is very important to understand the difference between a class and the objects or instances generated through its usage. Object-oriented programming allows us to discover the blueprint we used to generate a specific object. Thus, we are able to infer that each object is an instance of the rectangle class.
Now, we need to design the classes to include the attributes that provide the required data to each instance. In other words, we have to make sure that each class has the necessary variables that encapsulate all the data required by the objects to perform all the tasks.
Let's start with the Square class. It is necessary to know the length of the sides for each instance of this class - for each square object. Therefore, we need an encapsulated variable that allows each instance of this class to specify the value of the length of a side.
The variables defined in a class to encapsulate data for each instance of the class are known as attributes or fields. Each instance has its own independent value for the attributes or fields defined in the class.
The Square class defines a floating point attribute named LengthOfSide whose initial value is equal to 0 for any new instance of the class. After you create an instance of the Square class, it is possible to change the value of the LengthOfSide attribute.
For example, imagine that you create two instances of the Square class. One of the instances is named square1, and the other is square2. The instance names allow you to access the encapsulated data for each object, and therefore, you can use them to change the values of the exposed attributes.
Imagine that our object-oriented programming language uses a dot (.) to allow us to access the attributes of the instances. So, square1.LengthOfSide provides access to the length of side for the Square instance named square1, and square2.LengthOfSide does the same for the Square instance named square2.
You can assign the value 10 to square1.LengthOfSide and 20 to square2.LengthOfSide. This way, each Square instance is going to have a different value for their LengthOfSide attribute.
Now, let's move to the Rectangle class. We can define two floating-point attributes for this class: Width and Height. Their initial values are also going to be 0. Then, you can create two instances of the Rectangle class: rectangle1 and rectangle2.
You can assign the value 10 to rectangle1.Width and 20 to rectangle1.Height. This way, rectangle1 represents a 10 x 20 rectangle. You can assign the value 30 to rectangle2.Width and 50 to rectangle2.Height to make the second Rectangle instance representing a 30 x 50 rectangle.
Python, JavaScript, and C# support object-oriented programming, also known as OOP. However, each programming language takes a different approach. Both Python and C# support classes and inheritance. Therefore, you can use the different syntax provided by each of these programming languages to declare the Shape class and its four subclasses. Then, you can create instances of each of the subclasses and call the different methods.
On the other hand, JavaScript uses an object-oriented model that doesn't use classes. This object-oriented model is known as prototype-based programming. However, don't worry. Everything you have learned so far in your simple object-oriented design journey can be coded in JavaScript. Instead of using inheritance to achieve behavior reuse, we can expand upon existing objects. Thus, we can say that objects serve as prototypes in JavaScript. Instead of focusing on classes, we work with instances and decorate them to emulate inheritance in class-based languages.
The object-oriented model named prototype-based programing is also known with other names such as classless programming, instance-based programming, or prototype-oriented programming.
There are other important differences between Python, JavaScript, and C#. They have a great impact on the way you can code object-oriented designs.
Carry on reading Learning Object-Oriented Programming to learn different ways to code the same object-oriented design in three programming languages.
So what are you waiting for? Take a look at what else the book offers now!