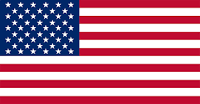
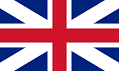
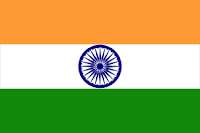
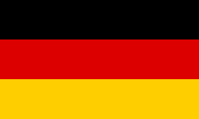
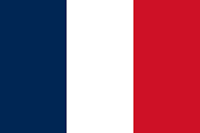
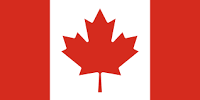
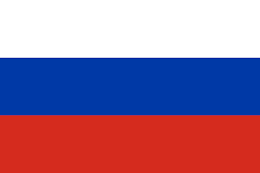
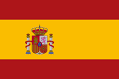
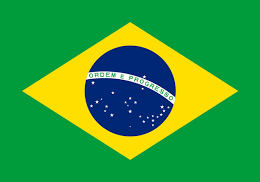
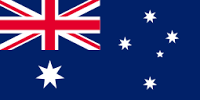
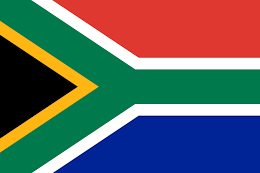
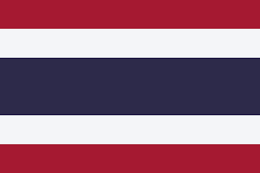
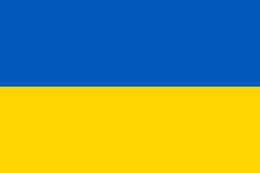
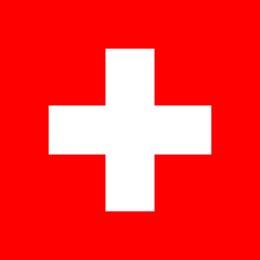
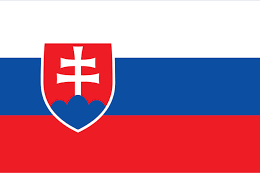
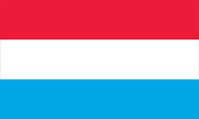
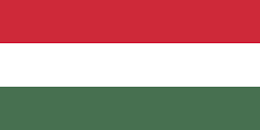
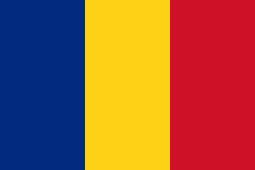
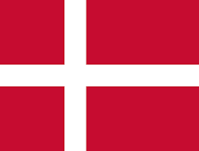
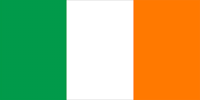
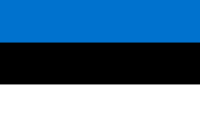
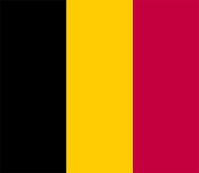
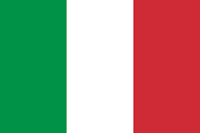
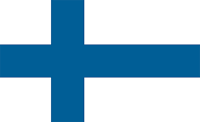
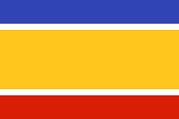
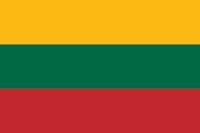
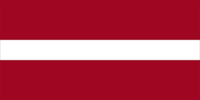
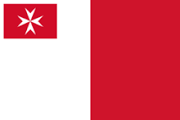
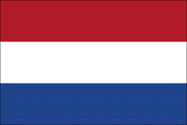
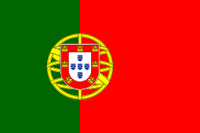
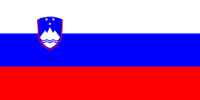
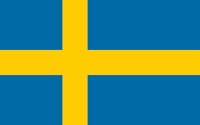
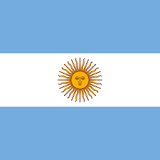
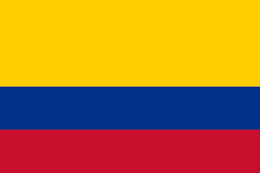
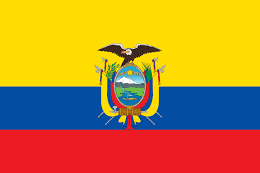
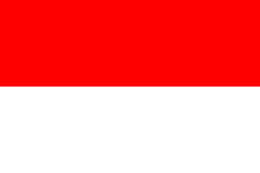
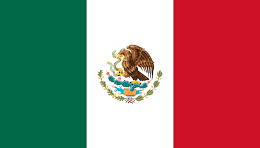
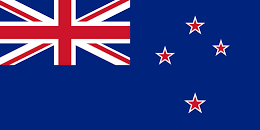
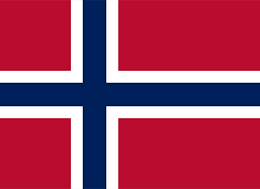
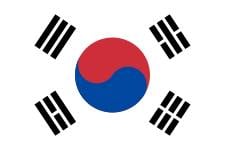
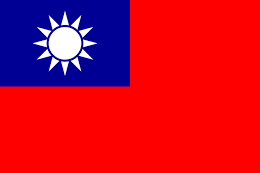
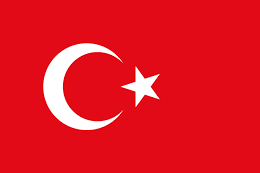
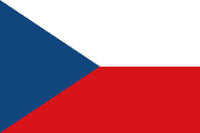
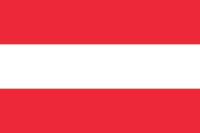
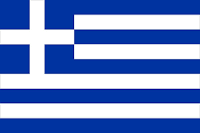
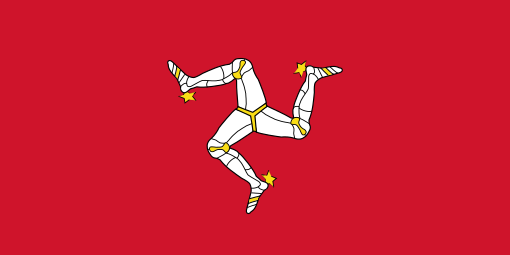
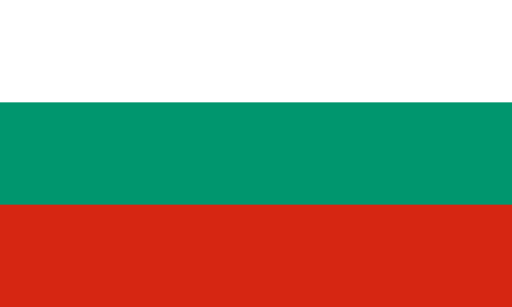
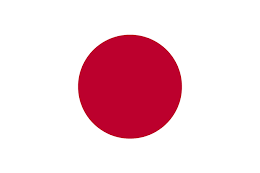
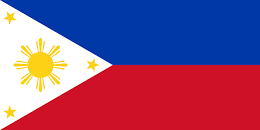
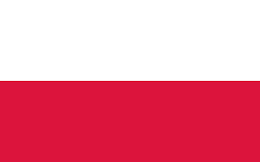

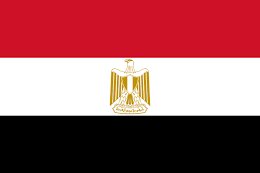
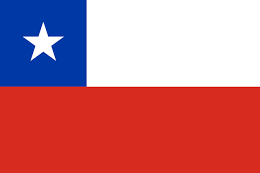
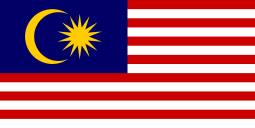
This recipe uses a login form as an example to explain how to create required fields in a form.
Ext.QuickTips.init();
var loginForm = { xtype: 'form',
id: 'login-form',
bodyStyle: 'padding:15px;
background:transparent',
border: false,
url:'login.php',
items: [{
xtype: 'box',
autoEl: { tag: 'div',
html: '<div class="app-msg">
<img src="img/magic-wand.png" class="app-img" />
Log in to The Magic Forum</div>'}
},
{ xtype: 'textfield',
id: 'login-user',
fieldLabel: 'Username',
allowBlank: false
},
{ xtype: 'textfield',
id: 'login-pwd',
fieldLabel: 'Password',
inputType: 'password',allowBlank: false
}],
buttons: [{
text: 'Login',
handler: function() {
Ext.getCmp('login-form').getForm().submit();
}
},
{
text: 'Cancel',
handler: function() {
win.hide();
}
}]
}
Ext.onReady(function() {
win = new Ext.Window({
layout: 'form',
width: 340,
autoHeight: true,
closeAction: 'hide',
items: [loginForm]
});
win.show();
});
Initializing the QuickTips singleton allows the form's validation errors to be shown as tool tips. When the form is created, each required field needs to have the allowblank configuration option set to false:
{ xtype: 'textfield', id: 'login-user', fieldLabel: 'Username',
allowBlank: false
},
{ xtype: 'textfield', id: 'login-pwd', fieldLabel: 'Password',
inputType: 'password', allowBlank: false
}
Setting allowBlank to false activates a validation rule that requires the length of the field's value to be greater than zero.
Use the blankText configuration option to change the error text when the blank validation fails. For example, the username field definition in the previous code snippet can be changed as shown here:
{ xtype: 'textfield', id: 'login-user', fieldLabel: 'Username',
allowBlank: false, blankText:'Enter your username'
}
The resulting error is shown in the following figure:
Validation rules can be combined and even customized. Other recipes in this article explain how to range-check a field's length, as well as how to specify the valid format of the field's value.
This recipe shows how to set the minimum and maximum number of characters allowed for a text field. The way to specify a custom error message for this type of validation is also explained.
The login form built in this recipe has username and password fields of a login form whose lengths are restricted:
Ext.QuickTips.init();
var loginForm = { xtype: 'form',
id: 'login-form',
bodyStyle: 'padding:15px;background:transparent',
border: false,
url:'login.php',
items: [
{ xtype: 'box',
autoEl: { tag: 'div',
html: '<div class="app-msg">
<img src="img/magic-wand.png" class="app-img" />
Log in to The Magic Forum</div>'
}
},
{ xtype: 'textfield',id: 'login-user',
fieldLabel: 'Username',
allowBlank: false,minLength: 3,maxLength: 32
},
{ xtype: 'textfield',id: 'login-pwd',
fieldLabel: 'Password',inputType: 'password',
allowBlank: false,minLength: 6,maxLength: 32,
minLengthText: 'Password must be at least 6 characters
long.'
}
],
buttons: [{
text: 'Login',
handler: function() {
Ext.getCmp('login-form').getForm().submit();
}
},
{
text: 'Cancel',
handler: function() {
win.hide();
}
}]
}
Ext.onReady(function() {
win = new Ext.Window({
layout: 'form',
width: 340,
autoHeight: true,
closeAction: 'hide',
items: [loginForm]
});
win.show();
});
After initializing the QuickTips singleton, which allows the form's validation errors to be shown as tool tips, the form is built.
The form is an instance of Ext.form.FormPanel. The username and password fields have their lengths restricted by the way of the minLength and maxLength configuration options:
{ xtype: 'textfield', id: 'login-user', fieldLabel: 'Username',
allowBlank: false, minLength: 3, maxLength: 32
},
{ xtype: 'textfield', id: 'login-pwd',
fieldLabel: 'Password', inputType: 'password',
allowBlank: false, minLength: 6, maxLength: 32,
minLengthText: 'Password must be at least 6 characters long.'
}
Notice how the minLengthText option is used to customize the error message that is displayed when the minimum length validation fails:
{ xtype: 'textfield', id: 'login-pwd',
fieldLabel: 'Password', inputType: 'password',
allowBlank: false, minLength: 6, maxLength: 32,
minLengthText: 'Password must be at least 6 characters long.'
}
As a last step, the window that will host the form is created and displayed.
You can also use the maxLengthText configuration option to specify the error message when the maximum length validation fails.