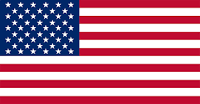
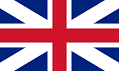
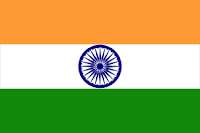
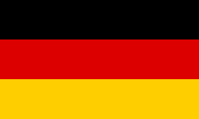
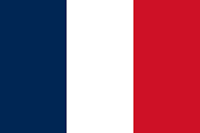
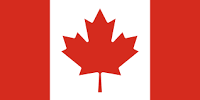
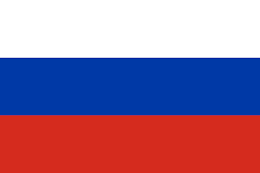
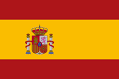
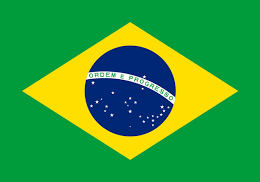
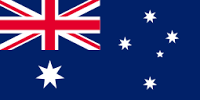
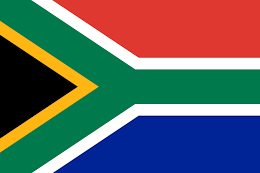
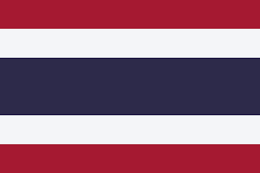
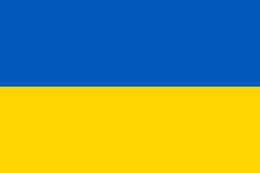
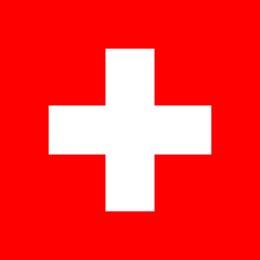
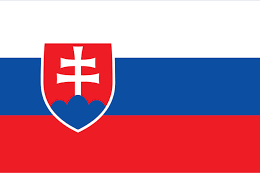
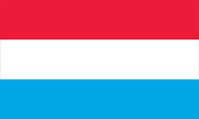
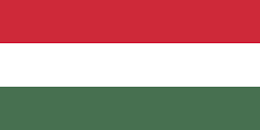
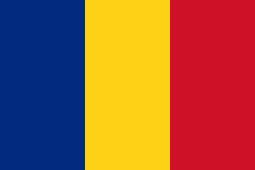
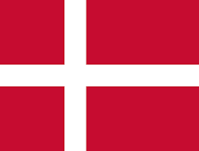
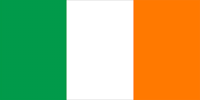
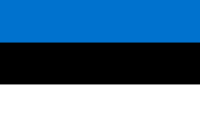
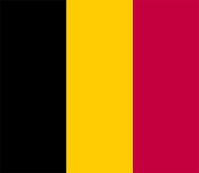
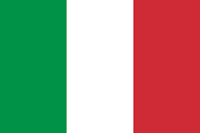
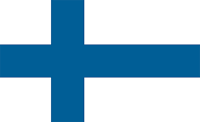
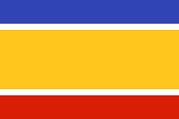
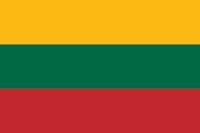
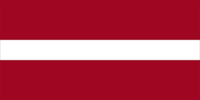
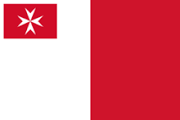
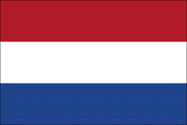
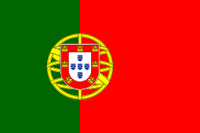
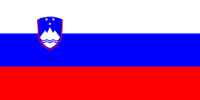
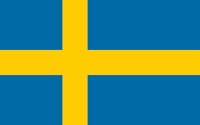
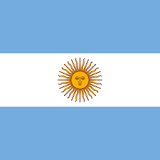
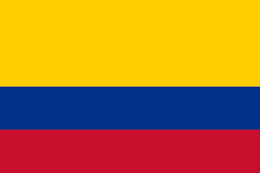
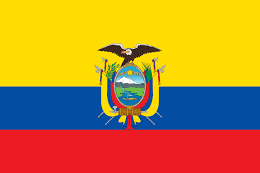
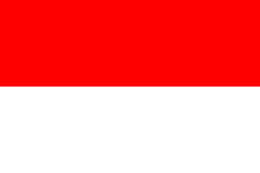
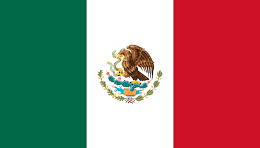
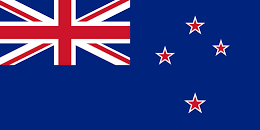
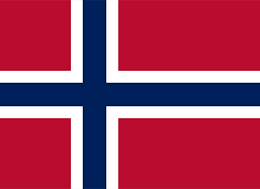
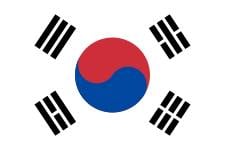
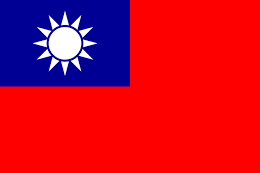
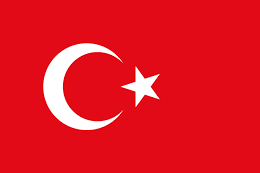
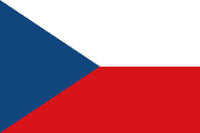
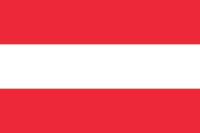
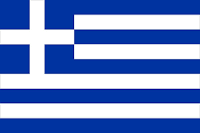
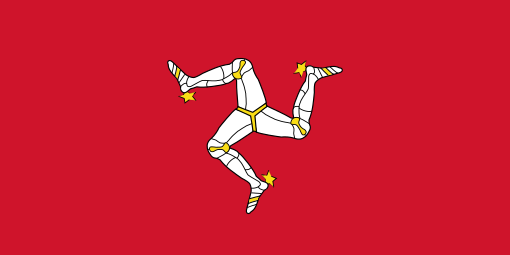
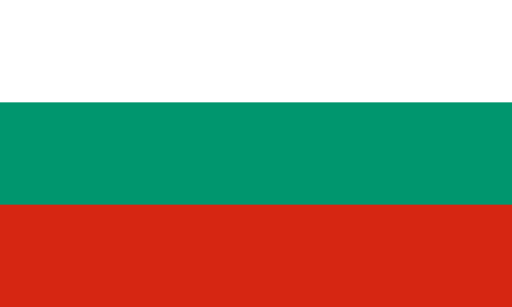
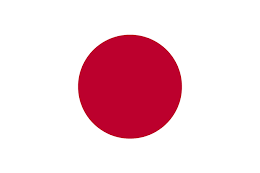
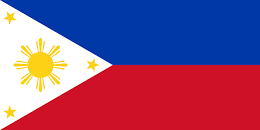
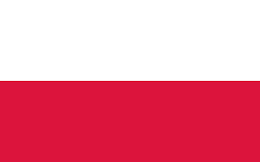

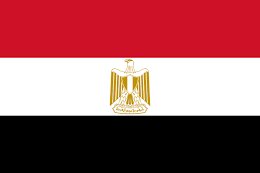
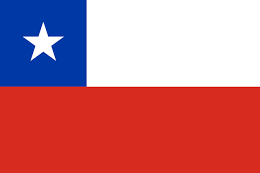
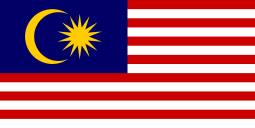
(For more resources on Alfresco, see here.)
For the first step, you will see how to set up the .NET project in the development environment. Then when we take a look at the sample code, we will learn how to perform the following operations from your .NET application:
In order to execute samples included with this article, you need to download and install the following software components in your Windows operating system:
The Microsoft .NET Framework 3.5 is the main framework used to compile the application, and you can download it using the following URL:
http://www.microsoft.com/downloads/details.aspx?familyid=333325fd-ae52-4e35-b531-508d977d32a6&displaylang=en.
Before importing the code in the development environment, you need to download and install the Web Services Enhancements (WSE) 3.0, which you can find at this address:
http://www.microsoft.com/downloads/details.aspx?FamilyID=018a09fd-3a74-43c5-8ec1-8d789091255d.
You can find more information about the Microsoft .NET framework on the official site at the following URL: http://www.microsoft.com/net/. From this page, you can access the latest news and the Developer Center where you can find the official forum and the developer community.
SharpDevelop 3.2 IDE is an open source IDE for C# and VB.NET, and you can download it using the following URL:
http://www.icsharpcode.net/OpenSource/SD/Download/#SharpDevelop3x.
Once you have installed all the mentioned software components, you can import the sample project into SharpDevelop IDE in the following way:
Now you should see a similar project tree in your development environment:
More information about SharpDevelop IDE can be found on the official site at the following address: http://www.icsharpcode.net/opensource/sd/. From this page, you can download different versions of the product; which SharpDevelop IDE version you choose depends on the .NET version which you would like to use. You can also visit the official forum to interact with the community of developers.
Also, notice that all the source code included with this article was implemented extending an existent open source project named dotnet. The dotnet project is available in the Alfresco Forge community, and it is downloadable from the following address:
http://forge.alfresco.com/projects/dotnet/.
Once you have set up the .NET solution in SharpDevelop, as explained in the previous section, you can execute all the tests to verify that the client is working correctly. We have provided a batch file named build.bat to allow you to build and run all the integration tests. You can find this batch file in the root folder of the sample code.
Notice that you need to use a different version of msbuild for each different version of the .NET framework. If you want to compile using the .NET Framework 3.5, you need to set the following path in your environment:
set PATH=%PATH%;%WinDir%Microsoft.NETFrameworkv3.5
Otherwise, you have to set .NET Framework 2.0 using the following path:
set PATH=%PATH%;%WinDir%Microsoft.NETFrameworkv2.0.50727
We are going to assume that Alfresco is running correctly and it is listening on host localhost and on port 8080. Once executed, the build.bat program should start compiling and executing all the integration tests included in this article. After a few seconds have elapsed, you should see the following output in the command line:
...
...
...
*****************
* Running tests *
*****************
NUnit version 2.5.5.10112
Copyright (C) 2002-2009 Charlie Poole.
Copyright (C) 2002-2004 James W. Newkirk, Michael C. Two, Alexei A.
Vorontsov.
Copyright (C) 2000-2002 Philip Craig.
All Rights Reserved.
Runtime Environment -
OS Version: Microsoft Windows NT 5.1.2600 Service Pack 2
CLR Version: 2.0.50727.3053 ( Net 2.0 )
ProcessModel: Default DomainUsage: Single
Execution Runtime: net-2.0
............
Tests run: 12, Errors: 0, Failures: 0, Inconclusive: 0, Time: 14.170376
seconds
Not run: 0, Invalid: 0, Ignored: 0, Skipped: 0
********
* Done *
********
As you can see from the project tree, you have some of the following packages:
The Search package shows you how to perform queries against the repository.
The Crud package contains samples related to all the CRUD operations that show you how to perform basic operations; namely, how to create/get/update/remove nodes in the repository.
The Association package shows you how to create and remove association instances among nodes.
Once you have authenticated a user, you can start to execute queries against the repository. In the following sample code, we will see how to perform a query using the RepositoryService of Alfresco:
RepositoryService repositoryService = WebServiceFactory.
getRepositoryService();
Then we need to create a store where we would like to search contents:
Store spacesStore = new Store(StoreEnum.workspace, "SpacesStore");
Now we need to create a Lucene query. In this sample, we want to search the Company Home space, and this means that we have to execute the following query:
String luceneQuery = "PATH:"/app:company_home"";
In the next step, we need to use the query method available from the RepositoryService. In this way, we can execute the Lucene query and we can get all the results from the repository:
Query query =
new Query(Constants.QUERY_LANG_LUCENE, luceneQuery);QueryResult queryResult =
repositoryService.query(spacesStore, query, false);
You can retrieve all the results from the queryResult object, iterating the ResultSetRow object in the following way:
ResultSet resultSet = queryResult.resultSet;
ResultSetRow[] results = resultSet.rows;
//your custom list
IList<CustomResultVO> customResultList =
new List<CustomResultVO>();
//retrieve results from the resultSet
foreach(ResultSetRow resultRow in results)
{
ResultSetRowNode nodeResult = resultRow.node;
//create your custom value object
CustomResultVO customResultVo = new CustomResultVO();
customResultVo.Id = nodeResult.id;
customResultVo.Type = nodeResult.type;
//retrieve properties from the current node
foreach(NamedValue namedValue in resultRow.columns)
{
if (Constants.PROP_NAME.Equals(namedValue.name))
{
customResultVo.Name = namedValue.value;
} else if (Constants.PROP_DESCRIPTION.Equals(namedValue.name))
{
customResultVo.Description = namedValue.value;
}
}
//add the current result to your custom list
customResultList.Add(customResultVo);
}
In the last sample, we iterated all the results and we created a new custom list with our custom value object CustomResultVO.
More information about how to build Lucene queries can be found at this URL: http://wiki.alfresco.com/wiki/Search.
We can perform various operations on the repository. They are documented as follows:
For each operation, you need to authenticate users before performing all the required operations on nodes. The class that provides the authentication feature is named AuthenticationUtils, and it allows you to invoke the startSession and endSession methods:
String username = "johndoe";
String password = "secret";
AuthenticationUtils.startSession(username, password);
try{
}
finally
{
AuthenticationUtils.endSession();
}
Remember that the startSession method requires the user credentials: the username as the first argument and the password as the second.
Notice that the default endpoint address of the Alfresco instance is as follows:
http://localhost:8080/alfresco
If you need to change the endpoint address, you can use the WebServiceFactory class invoking the setEndpointAddress method to set the new location of the Alfresco repository.