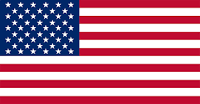
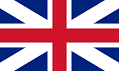
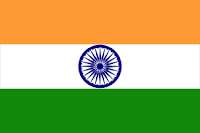
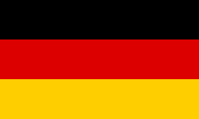
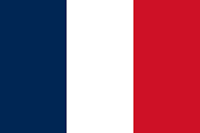
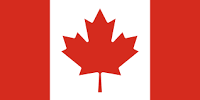
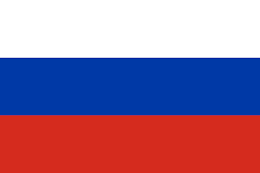
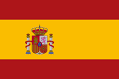
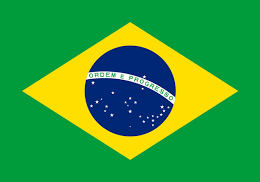
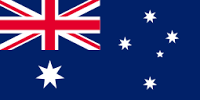
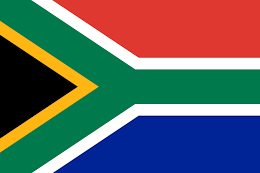
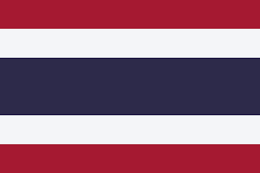
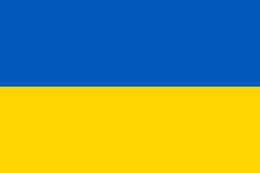
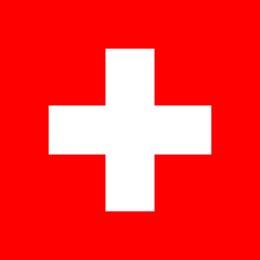
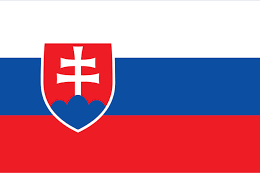
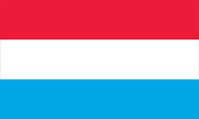
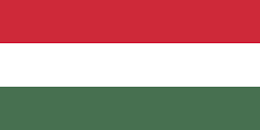
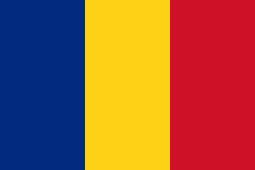
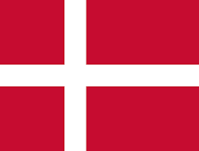
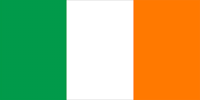
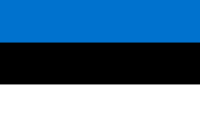
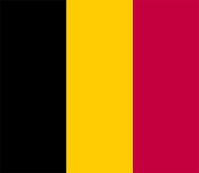
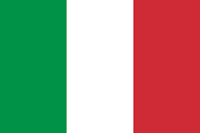
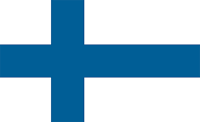
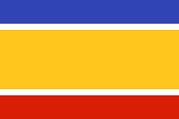
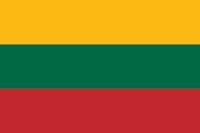
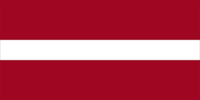
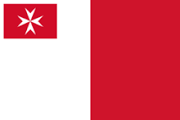
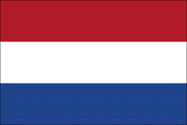
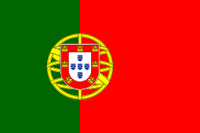
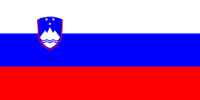
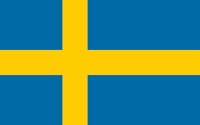
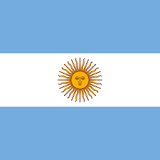
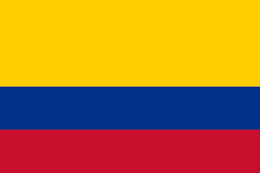
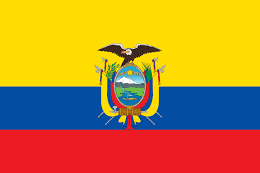
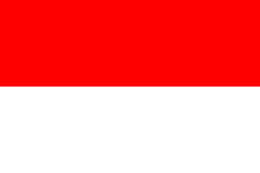
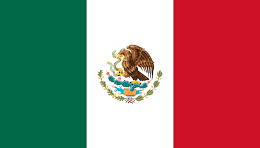
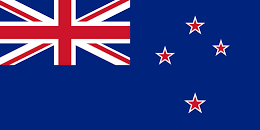
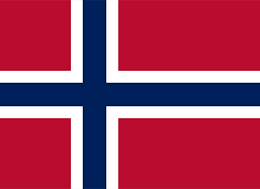
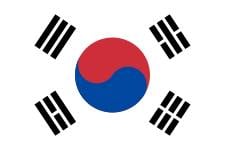
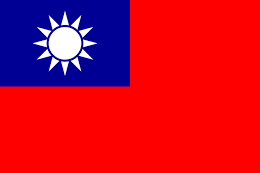
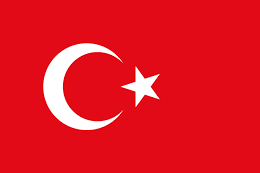
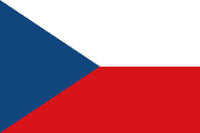
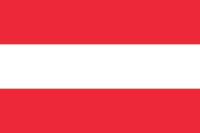
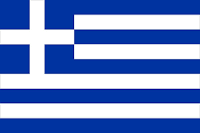
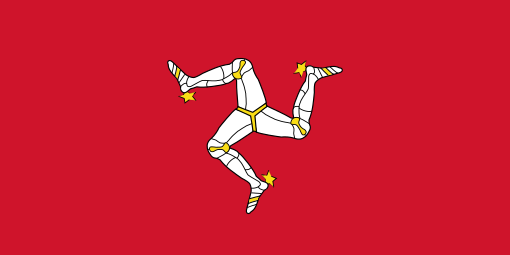
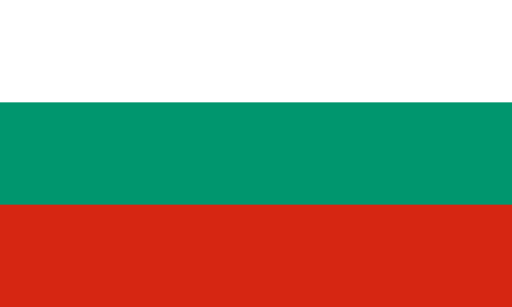
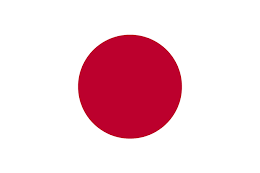
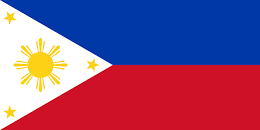
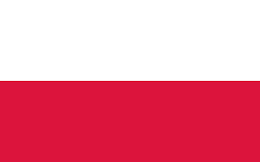

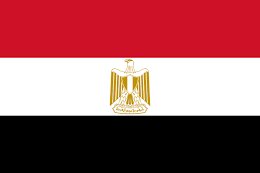
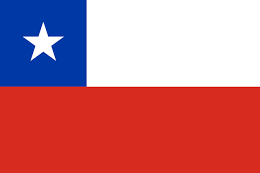
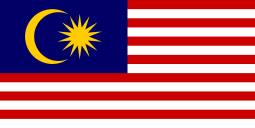
Over 70 simple but incredibly effective practical recipes to develop web applications using GWT with JPA , MySQL and i Report
The Graphical User Interface (GUI) resides in the client side of the application. This article introduces the communication between the server and the client, where the client (GUI) will send a request to the server, and the server will respond accordingly. In GWT, the interaction between the server and the client is made through the RPC mechanism. RPC stands for Remote Procedure Call. The concept is that there are some methods in the server side, which are called by the client at a remote location. The client calls the methods by passing the necessary arguments, and the server processes them, and then returns back the result to the client. GWT RPC allows the server and the client to pass Java objects back and forth.
RPC has the following steps:
In this application, the server and the client will pass Java objects back and forth for the operation. For example, the BranchForm will request the server to persist a Branch object, where the Branch object is created and passed to server by the client, and the server persists the object in the server database. In another example, the client will pass the Branch ID (as an int), the server will find the particular Branch information, and then send the Branch object to the client to be displayed in the branch form. So, both the server and client need to send or receive Java objects. We have already created the JPA entity classes and the JPA controller classes to manage the entity using the Entity Manager. But the JPA class objects are not transferable over the network using the RPC. JPA classes will just be used by the server on the server side. For the client side (to send and receive objects), DTO classes are used. DTO stands for Data Transfer Object. DTO is simply a transfer object which encapsulates the business data and transfers it across the network.
Create a package com.packtpub.client.dto, and create all the DTO classes in this package.
The steps required to complete the task are as follows:
public class BranchDTO implements Serializable
private Integer branchId;
private String name;
private String location
public BranchDTO(Integer branchId, String name, String location)
{
this.branchId = branchId;
this.name = name;
this.location = location;
}
public BranchDTO(Integer branchId, String name)
{
this.branchId = branchId;
this.name = name;
}
public BranchDTO(Integer branchId)
{
this.branchId = branchId;
}
public BranchDTO()
{
}
To generate the constructors automatically in NetBeans, right-click on the code, select Insert Code | Constructor, and then click on Generate after selecting the attribute(s).
public Integer getBranchId()
{
return branchId;
}
public void setBranchId(Integer branchId)
{
this.branchId = branchId;
}
public String getLocation()
{
return location;
}
public void setLocation(String location)
{
this.location = location;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
To generate the setter and getter automatically in NetBeans, right-click on the code, select Insert Code | Getter and Setter…, and then click on Generate after selecting the attribute(s).
In RPC, the client will send and receive DTOs, but the server needs pure JPA objects to be used by the Entity Manager. That's why, we need to transform from DTO to JPA entity class and vice versa. In this recipe, we will learn how to map the entity class and DTO.
Create the entity and DTO classes.
public Branch(BranchDTO branchDTO)
{
setBranchId(branchDTO.getBranchId());
setName(branchDTO.getName());
setLocation(branchDTO.getLocation());
}
Some third-party libraries are available for automatically mapping entity class and DTO, such as Dozer and Gilead. For details, you may visit http://dozer.sourceforge.net/ and http://noon.gilead.free.fr/gilead/.
In this recipe, we are going to create the GWTService interface, which will contain an abstract method to add a Branch object to the database.
Create the Branch entity class and the DTO class.