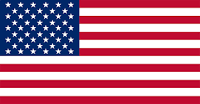
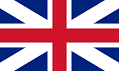
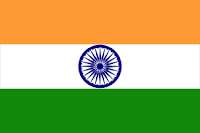
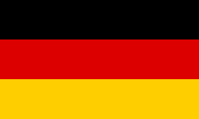
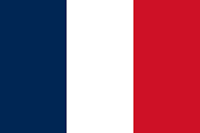
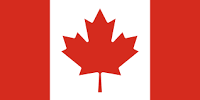
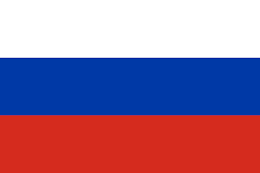
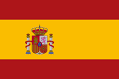
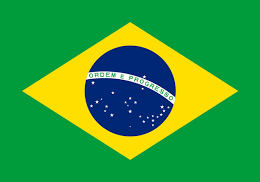
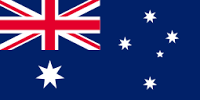
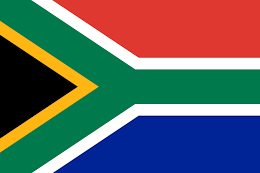
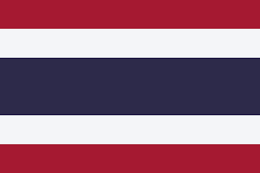
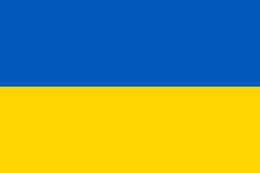
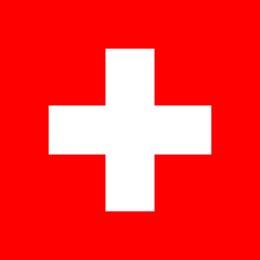
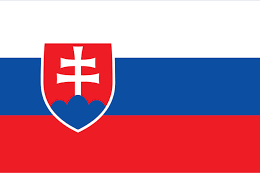
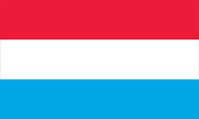
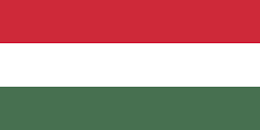
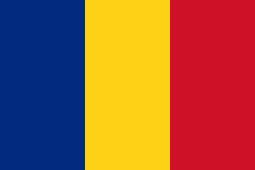
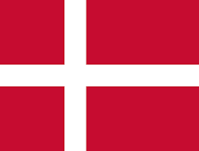
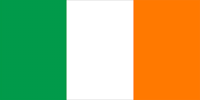
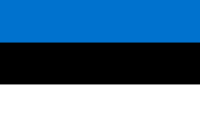
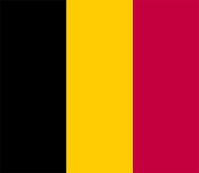
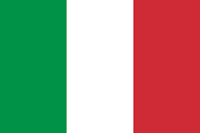
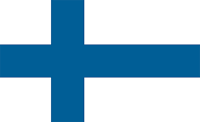
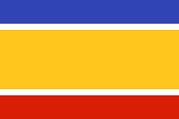
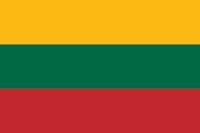
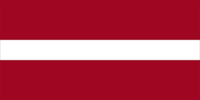
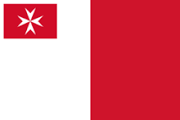
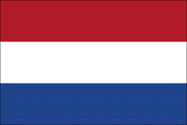
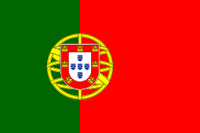
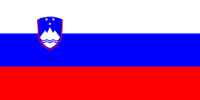
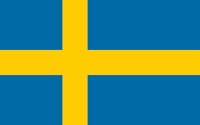
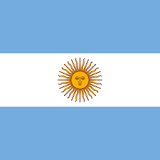
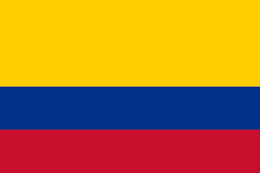
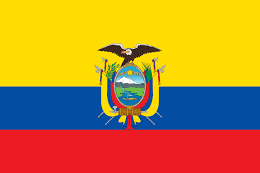
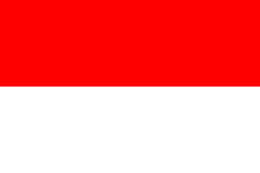
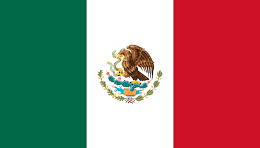
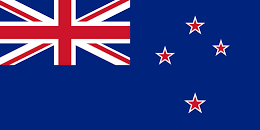
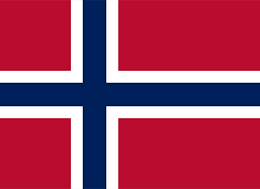
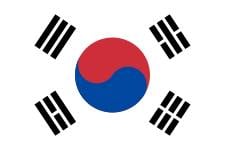
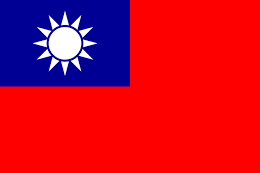
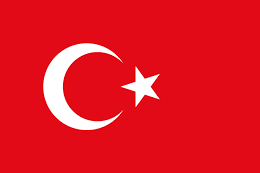
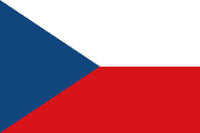
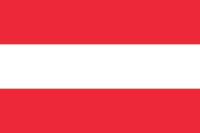
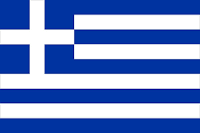
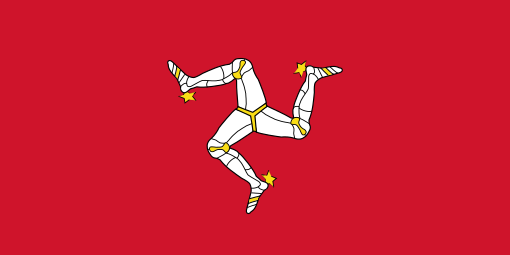
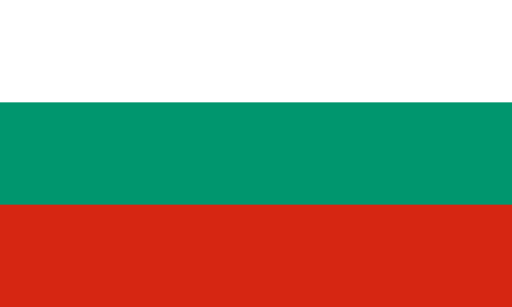
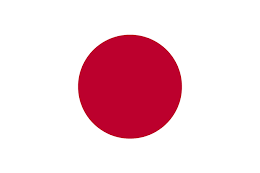
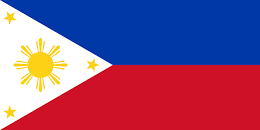
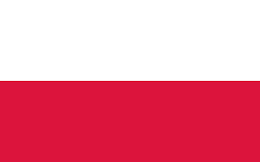

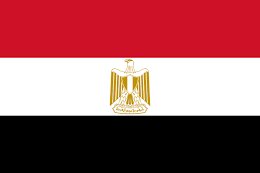
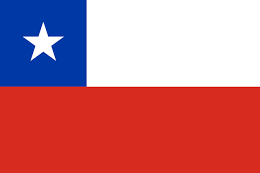
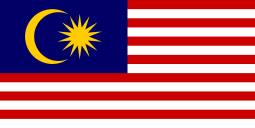
This article by Jason Dentler, author of NHibernate 3.0 Cookbook, shows how we can set up the data access layer to wrap all data access in NHibernate transactions automatically.
Complete the Eg.Core model and mappings. Download code (ch:1)
public class DataAccessObject<T, TId>
where T : Entity<TId>
{
private readonly ISessionFactory _sessionFactory;
private ISession session
{
get
{
return _sessionFactory.GetCurrentSession();
}
}
public DataAccessObject(ISessionFactory sessionFactory)
{
_sessionFactory = sessionFactory;
}
public T Get(TId id)
{
return Transact(() => session.Get<T>(id));
}
public T Load(TId id)
{
return Transact(() => session.Load<T>(id));
}
public void Save(T entity)
{
Transact(() => session.SaveOrUpdate(entity));
}
public void Delete(T entity)
{
Transact(() => session.Delete(entity));
}
private TResult Transact<TResult>(Func<TResult> func)
{
if (!session.Transaction.IsActive)
{
// Wrap in transaction
TResult result;
using (var tx = session.BeginTransaction())
{
result = func.Invoke();
tx.Commit();
}
return result;
}
// Don't wrap;
return func.Invoke();
}
private void Transact(Action action)
{
Transact<bool>(() =>
{
action.Invoke();
return false;
});
}
}
public class DataAccessObject<T>
: DataAccessObject<T, Guid>
where T : Entity
{
}
NHibernate requires that all data access occurs inside an NHibernate transaction and this can be easily accomplished with AOP.
Remember, the ambient transaction created by TransactionScope is not a substitute for an NHibernate transaction.
This recipe shows a more explicit approach. To ensure that at least all our data access layer calls are wrapped in transactions, we create a private Transact function that accepts a delegate, consisting of some data access methods, such as session.Save or session.Get. This Transact function first checks if the session has an active transaction. If it does, Transact simply invokes the delegate. If it doesn't, it creates an explicit NHibernate transaction, then invokes the delegate, and finally commits the transaction. If the data access method throws an exception, the transaction will be rolled back automatically as the exception bubbles up through the using block.
This transactional auto-wrapping can also be set up using SessionWrapper from the unofficial NHibernate AddIns project at http://code.google.com/p/unhaddins. This class wraps a standard NHibernate session. By default, it will throw an exception when the session is used without an NHibernate transaction. However, it can be configured to check for and create a transaction automatically, much in the same way I've shown you here.