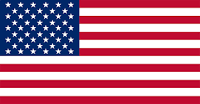
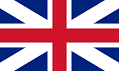
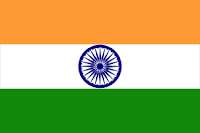
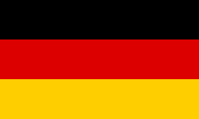
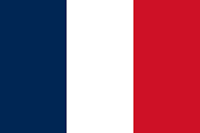
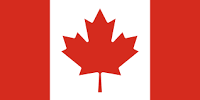
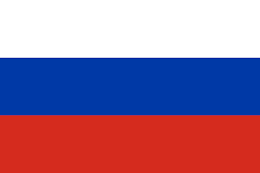
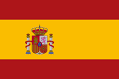
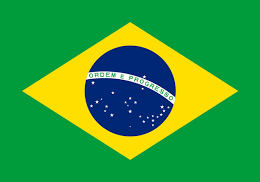
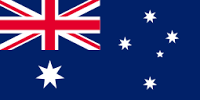
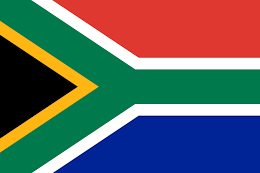
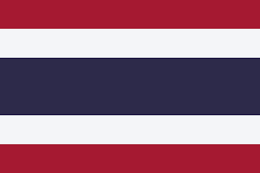
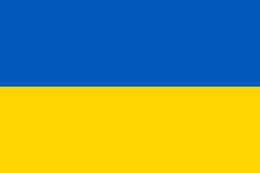
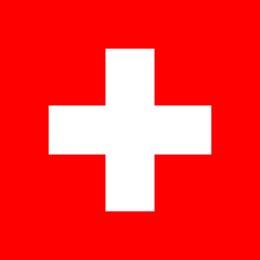
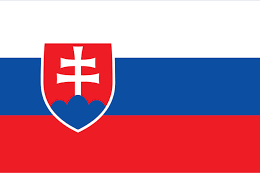
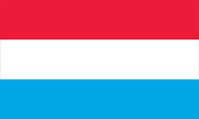
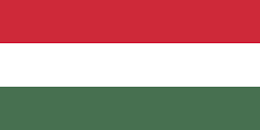
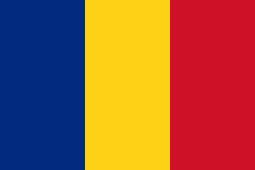
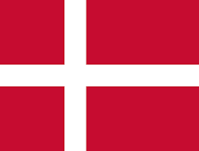
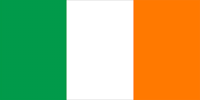
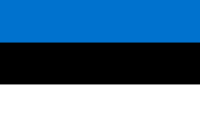
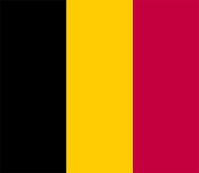
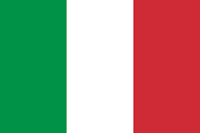
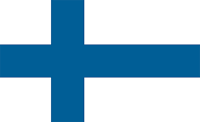
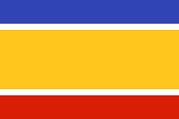
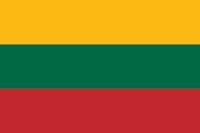
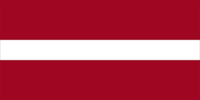
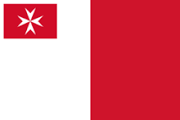
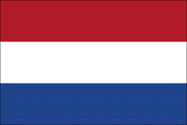
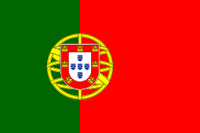
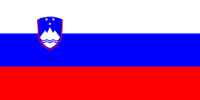
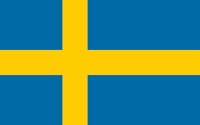
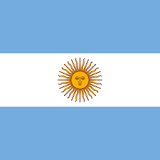
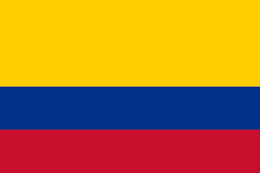
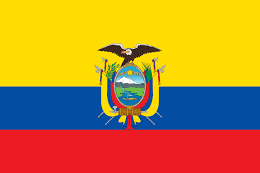
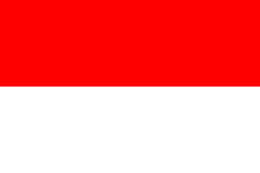
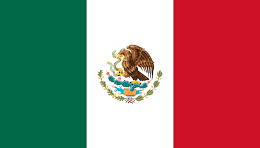
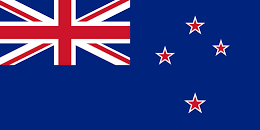
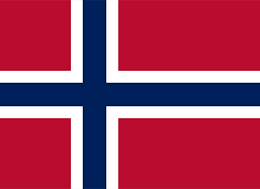
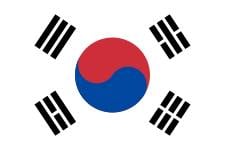
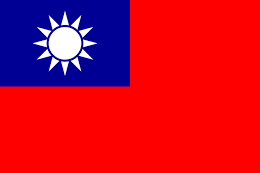
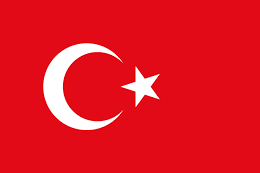
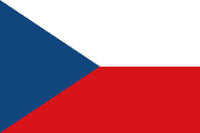
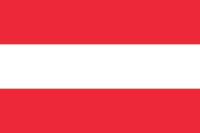
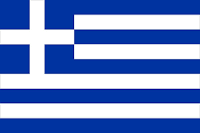
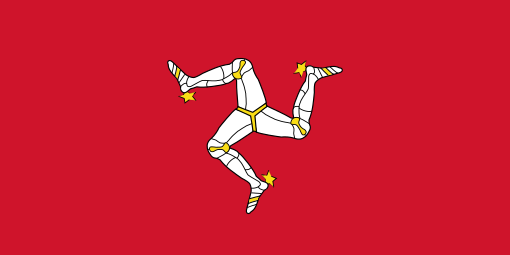
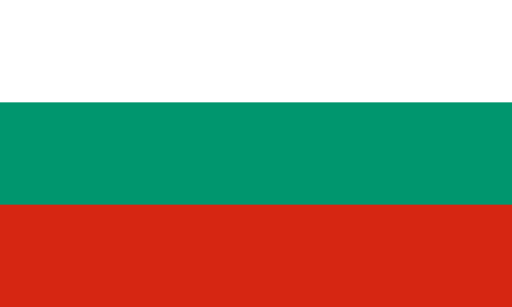
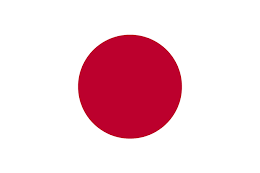
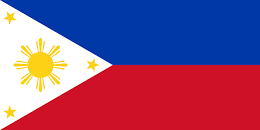
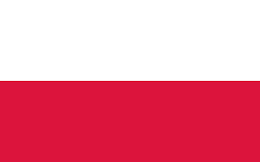

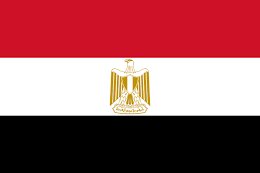
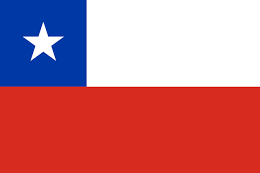
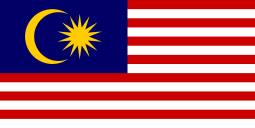
Get solutions to common NHibernate problems to develop high-quality performance-critical data access applications
The reader would benefit from reading the previous article on Testing Using NHibernate Profiler and SQLite.
Mappings are a critical part of any NHibernate application. In this recipe, I'll show you how to test those mappings using Fluent NHibernate's Persistence tester.
Complete the Fast testing with SQLite in-Memory database recipe mentioned in the previous article.
using FluentNHibernate.Testing;
[Test]
public void Product_persistence_test()
{
new PersistenceSpecification<Product>(Session)
.CheckProperty(p => p.Name, "Product Name")
.CheckProperty(p => p.Description, "Product Description")
.CheckProperty(p => p.UnitPrice, 300.85M)
.VerifyTheMappings();
}
[Test]
public void ActorRole_persistence_test()
{
new PersistenceSpecification<ActorRole>(Session)
.CheckProperty(p => p.Actor, "Actor Name")
.CheckProperty(p => p.Role, "Role")
.VerifyTheMappings();
}
[Test]
public void Movie_persistence_test()
{
new PersistenceSpecification<Movie>(Session)
.CheckProperty(p => p.Name, "Movie Name")
.CheckProperty(p => p.Description, "Movie Description")
.CheckProperty(p => p.UnitPrice, 25M)
.CheckProperty(p => p.Director, "Director Name")
.CheckList(p => p.Actors, new List<ActorRole>()
{
new ActorRole() { Actor = "Actor Name", Role = "Role" }
})
.VerifyTheMappings();
}
The Persistence tester in Fluent NHibernate can be used with any mapping method. It performs the following four steps:
At a minimum, each entity type should have a simple Persistence test, such as the one shown previously. More information about the Fluent NHibernate Persistence tester can be found on their wiki at http://wiki.fluentnhibernate.org/Persistence_specification_testing