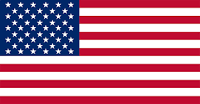
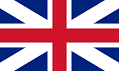
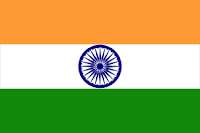
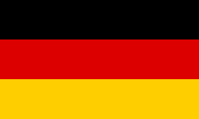
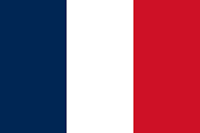
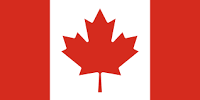
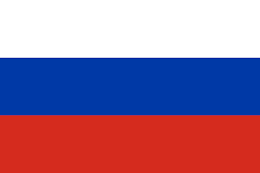
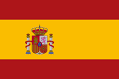
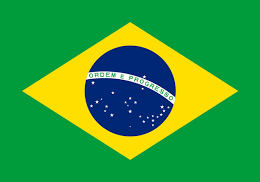
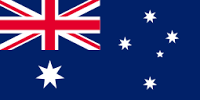
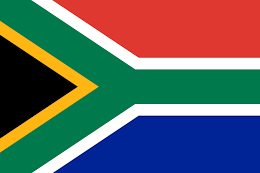
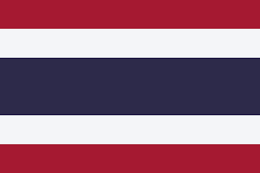
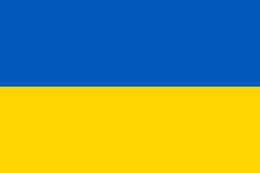
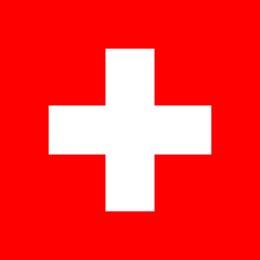
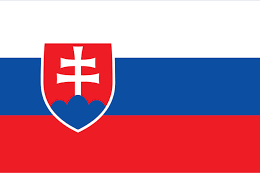
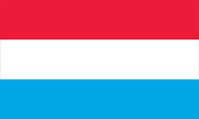
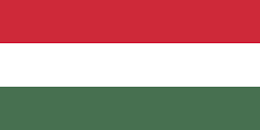
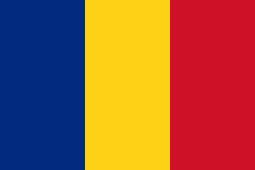
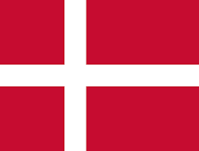
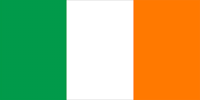
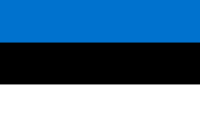
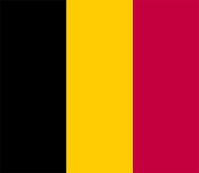
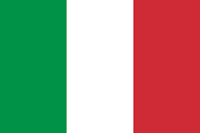
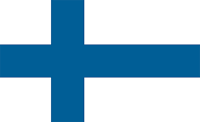
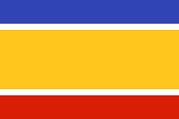
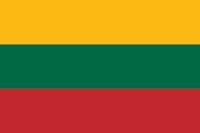
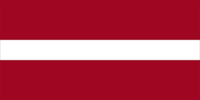
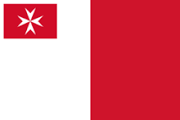
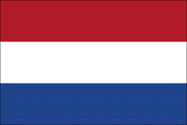
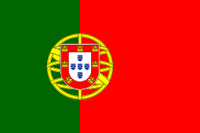
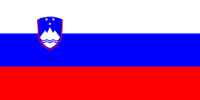
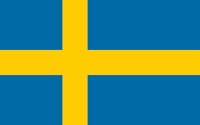
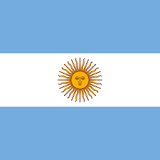
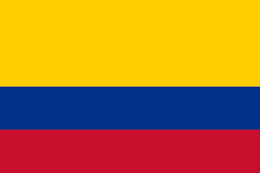
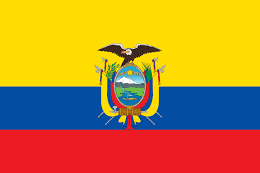
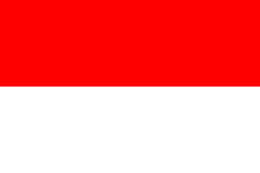
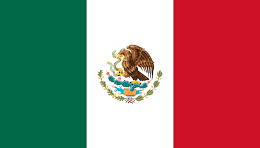
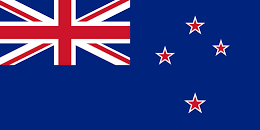
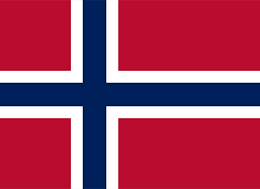
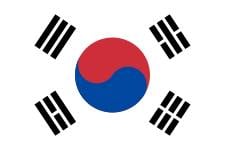
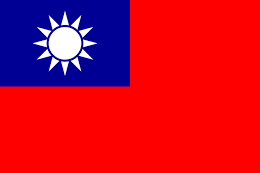
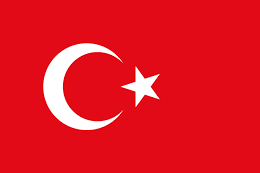
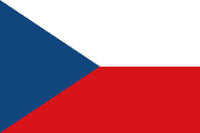
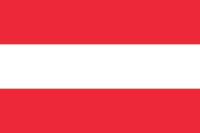
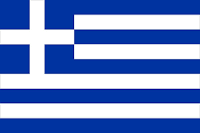
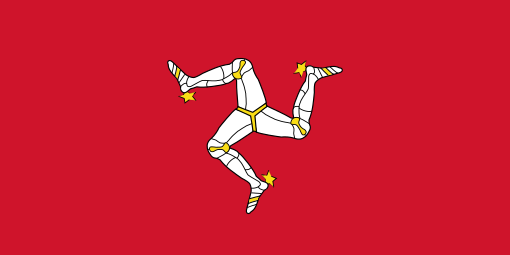
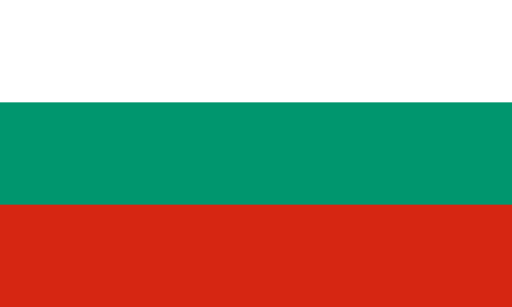
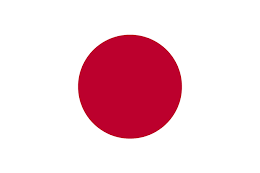
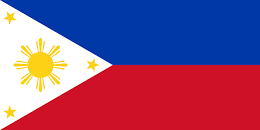
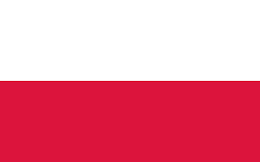

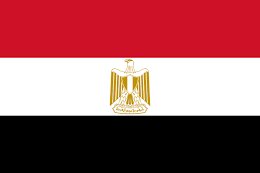
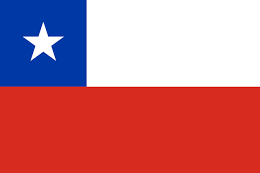
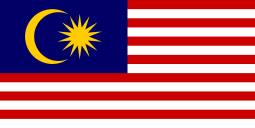
In-place editing means making the content available for editing just by clicking on it. We hover on the element, allow the user to click on the element, edit the content, and update the new content to our server.
Sounds complex? Not at all! It's very simple. Check out the example about www.netvibes.com shown in the following screenshot. You will notice that by just clicking on the title, we can edit and update it.
Now, check out the following screenshot to see what happens when we click on the title.
In simple terms, in-place editing is about converting the static content into an editable form without changing the place and updating it using AJAX.
Imagine that we can edit the content inside the static HTML tags such as a simple <p> or even a complex <div>.
The basic syntax of initiating the constructor is shown as follows:
New Ajax.InPlaceEditor(element,url,[options]);
The constructor accepts three parameters:
We shall look into the details of element and url in the next section. For now, let's learn about all the options that we will be using in our future examples.
The following set of options is provided by the script.aculo.us library. We can use the following options with the InPlaceEditor object:
We also have some callback options to use along with in-place editing.
We will be exploring all these options in our hands-on examples.
Now things are simple from here on. Let's get started with code.
First, let's include all the required scripts for in-place editing:
<script type="text/javascript" src="src/prototype.js"></script>
<script type="text/javascript" src="src/scriptaculous.js"></script>
<script type="text/javascript" src="src/effects.js"></script>
<script type="text/javascript" src="src/controls.js"></script>
Once this is done, let's create a basic HTML page with some <p> and <div> elements, and add some content to them.
<body>
<div id="myDiv">
First move the mouse over me and then click on ME :)
</div>
</body>
In this section we will be learning about the options provided with the in-place editing feature. In the hands-on section we will be working with server-side scripts of handling data.
Now, it's turn to add some spicy JavaScript code and create the object for InPlaceEditor.
In the following piece of code we have passed the element ID as myDIV, a fake URL,and two options okText and cancelText:
Function makeEditable() {
new Ajax.InPlaceEditor(
'myDIV',
'URL',
{
okText: 'Update',
cancelText: 'Cancel',
}
);
}
We will be placing them inside a function and we will call them on page load. So the complete script would look like this:
<script>
function makeEditable() {
new Ajax.InPlaceEditor(
'myDIV',
'URL',
{
okText: 'Update',
cancelText: 'Cancel'
}
);
}
</script>
<body onload="JavaScript:makeEditable();">
<div id="myDiv">
First move the mouse over me and then click on ME :)
</div>
</body>
Now, save the fi le as Inplace.html. Open it in a browser and you should see the result as shown in the following screenshot:
Now, let's add all the options step-by-step.
Remember, whatever we are adding now will be inside the definition of the constructor.
new Ajax.InPlaceEditor(
'myDIV',
'URL',
{
okText: 'Update',
cancelText: 'Cancel',
rows: 4,
cols: 70
}
);
new Ajax.InPlaceEditor(
'myDIV',
'URL',
{
okText: 'Update',
cancelText: 'Cancel',
rows: 4,
cols: 70,
highlightColor:'#E2F1B1'
}
);
new Ajax.InPlaceEditor(
'myDIV',
'URL',
{
okText: 'Update',
cancelText: 'Cancel',
rows: 4,
cols: 70,
highlightColor:'#E2F1B1',
clickToEditText: 'Click me to edit'
}
);